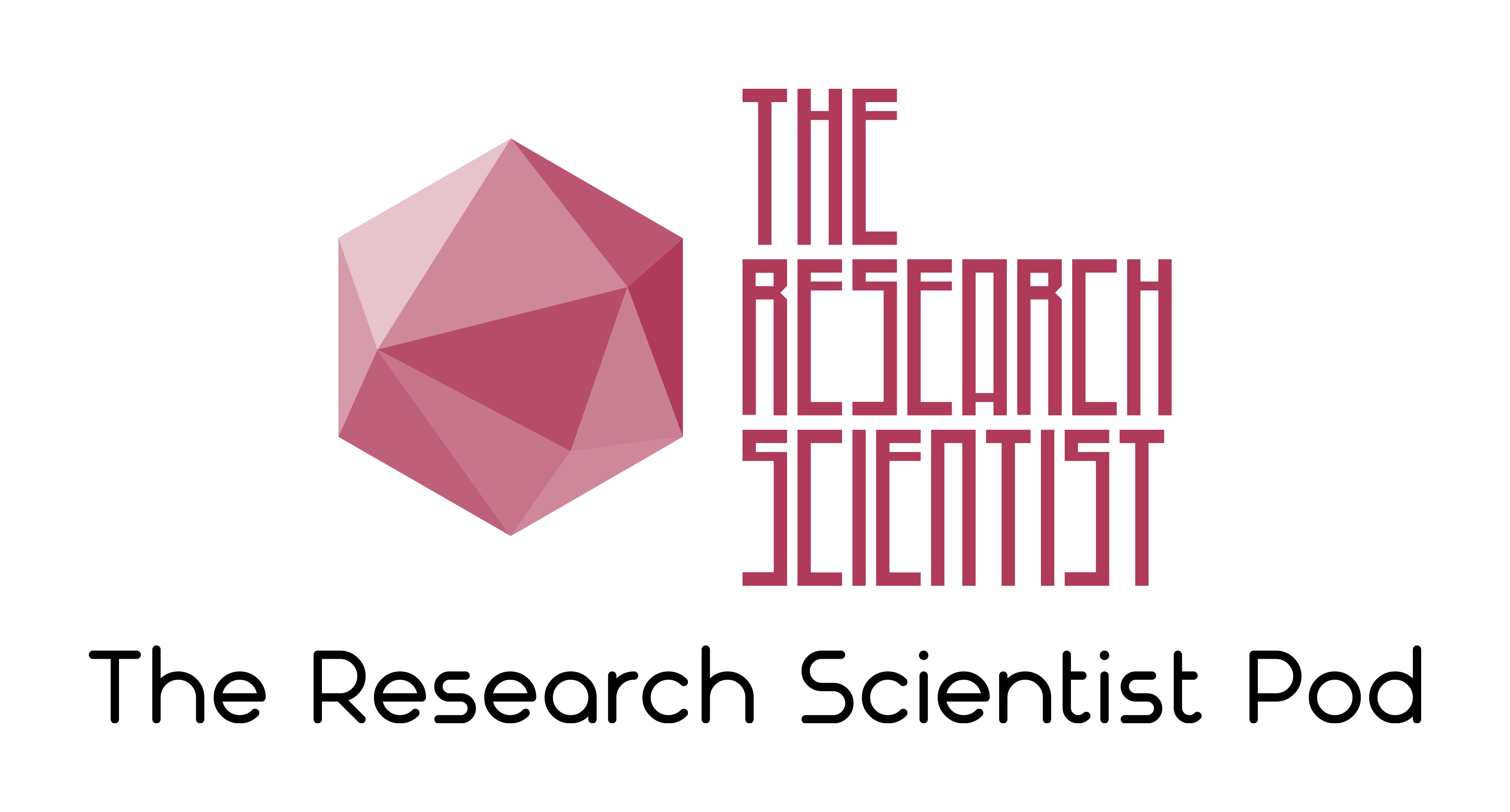
Enter two Z-scores to calculate the area under the normal distribution curve between them.
Area Between Z-Scores (P(Z1 ≤ Z ≤ Z2)):
Understanding the Area Between Two Z-Scores
The Z-Score, also known as the standard score, measures the number of standard deviations a data point is from the mean in a standard normal distribution (mean = 0, standard deviation = 1). This score is useful for comparing individual data points within the context of the entire distribution.
In statistics, the area between two Z-scores represents the probability that a random variable falls within the specified range. This area is often used to determine the likelihood of values within a certain interval, making it valuable for applications in fields like psychology, finance, and research for analyzing probability ranges.
Key Components of the Z-Score
- Z-Score: Indicates how many standard deviations a particular value is from the mean. Positive Z-scores are above the mean, while negative Z-scores are below the mean.
- Standard Normal Distribution: A normal distribution with a mean of 0 and a standard deviation of 1, used as the basis for calculating Z-scores and their probabilities.
Formula for the Standard Normal Distribution
The probability density function (PDF) for the standard normal distribution is:
Programmatically Calculating the Area Between Two Z-Scores
To calculate the area between two Z-scores, we can use popular libraries in JavaScript, Python, and R. Here’s how to perform these calculations:
1. Using JavaScript
In JavaScript, you can use the jStat library to calculate the area between two Z-scores.
// Calculate area between two Z-scores
const z1 = -1; // Lower Z-score
const z2 = 1; // Upper Z-score
// CDF: P(Z1 ≤ Z ≤ Z2)
const areaBetween = jStat.normal.cdf(z2, 0, 1) - jStat.normal.cdf(z1, 0, 1);
console.log('Area Between (P(-1 ≤ Z ≤ 1)):', areaBetween);
Note: Ensure you have included the jStat library in your project to perform these calculations.
2. Using Python
In Python, the SciPy library provides functions for cumulative distribution calculations.
from scipy.stats import norm
# Define the Z-scores
z1 = -1 # Lower Z-score
z2 = 1 # Upper Z-score
# CDF: P(Z1 ≤ Z ≤ Z2)
area_between = norm.cdf(z2) - norm.cdf(z1)
print("Area Between (P(-1 ≤ Z ≤ 1)):", area_between)
Note: Install SciPy by running pip install scipy
if it’s not already installed.
3. Using R
In R, the stats package includes functions for cumulative probability calculations for the normal distribution.
# Define the Z-scores
z1 <- -1 # Lower Z-score
z2 <- 1 # Upper Z-score
# CDF: P(Z1 ≤ Z ≤ Z2)
area_between <- pnorm(z2) - pnorm(z1)
cat("Area Between (P(-1 ≤ Z ≤ 1)):", area_between, "\n")
Note: The pnorm
function in R calculates the cumulative probability to the left of each Z-score, so we subtract to find the area between them.
Example Calculation
Let's assume we have Z-scores of \( z_1 = -1 \) and \( z_2 = 1 \). The area between these Z-scores, or \( P(-1 \leq Z \leq 1) \), represents the probability that a randomly chosen value from the distribution falls between -1 and 1 standard deviations from the mean.