Introduction
Binary Search is a cornerstone algorithm in computer science, widely used for efficiently searching elements in sorted collections. Its simplicity and power make it a go-to solution for countless problems involving large datasets.
In this blog post, we’ll dive into how Binary Search works and walk through its Python, C++, Java, and JavaScript implementations. We’ll explore its pseudocode, time and space complexity, practical use cases, application to different data structures, edge cases and pitfalls, and situations where Binary Search isn’t the best choice. Finally, we’ll look at key optimizations and variations to help you master this essential algorithm.
What Is Binary Search?
At its core, Binary Search is a “divide and conquer” algorithm that efficiently searches for a target element in a sorted collection (such as an array or list). The principle behind the algorithm is quite simple: it repeatedly divides the search space in half, systematically narrowing down the possible location of the target element.
Instead of scanning through each element individually, as you would in a linear search (with a time complexity of O(n), Binary Search eliminates half of the remaining elements in each step. This allows it to locate an element in a fraction of the time.
Binary Search: Divide and Conquer
Binary Search works by following this basic process:
- Start with the middle element: The algorithm begins by selecting the middle element of the array. This element serves as a checkpoint to decide whether the target value lies to the left or right of it.
- Compare the target with the middle element:
- If the middle element is the target, the search is complete.
- If the middle element is greater than the target, we discard the right half of the array and continue searching in the left half.
- If the middle element is less than the target, we discard the left half of the array and search for the right half.
- Repeat the process: Continue dividing the remaining search space in half until the target is found or the search space is exhausted.
Below is an interactive Binary Search Visualizer that lets you see how the algorithm operates in real-time.
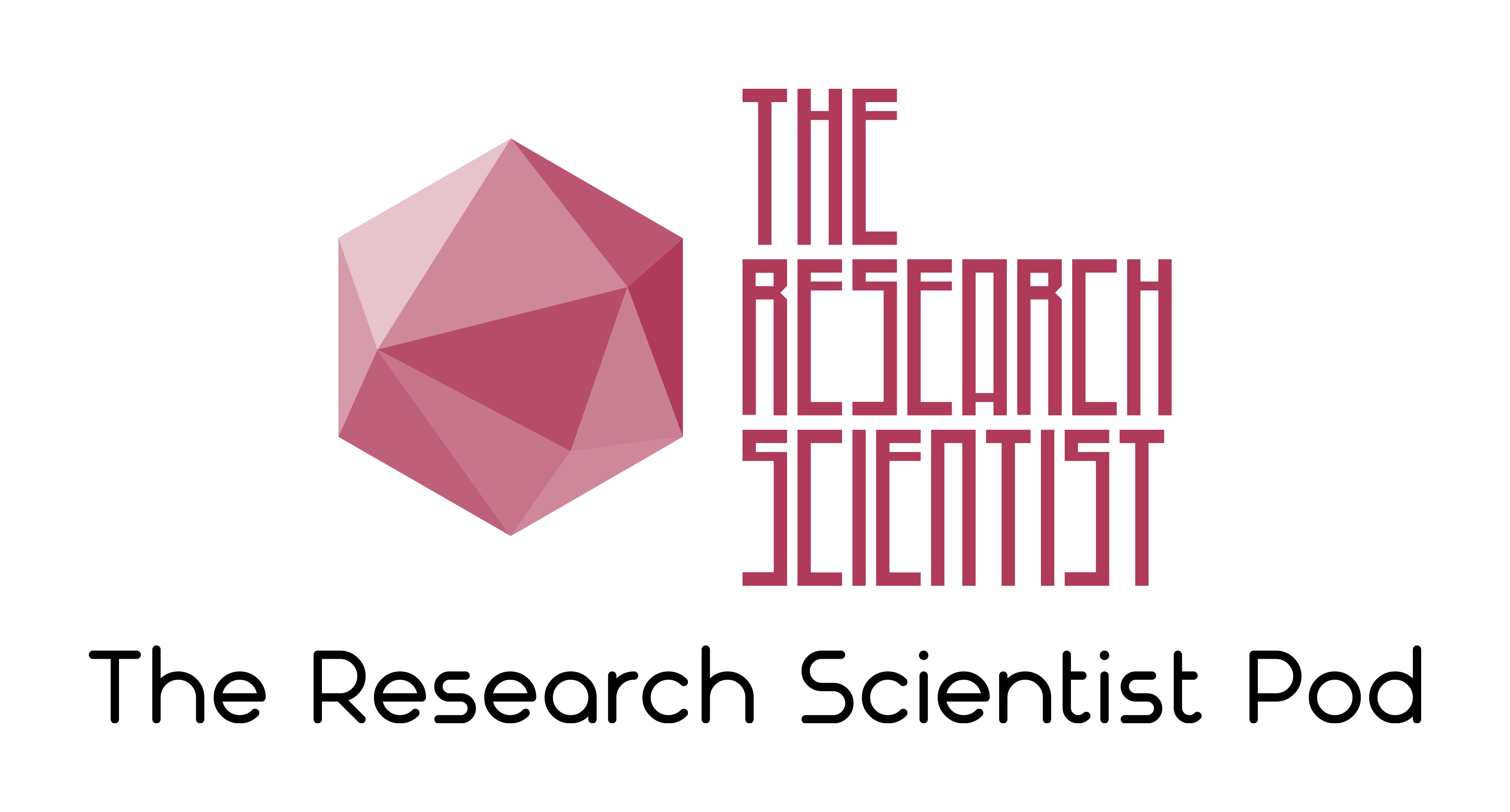
Analogy for Binary Search: Looking for a Word in a Dictionary
Imagine you are trying to find a specific word in a dictionary, such as “penguin.” Instead of starting from the first page and flipping through every page until you find it (like linear search), you can use a more intelligent method—binary search, which follows a divide-and-conquer approach.
Here’s how it works:
- Open the dictionary to the middle page. You look at the word in the middle of the dictionary. If this word is “octopus,” and you’re searching for “penguin,” you now know that “penguin” comes after “octopus” because “p” comes after “o” in the alphabet.
- Ignore the first half of the dictionary. Since you know “penguin” must be in the second half of the dictionary, you completely ignore the first half (from A to O). This is a key part of divide and conquer—you’ve just reduced the problem size by half.
- Repeat with the remaining half. Now, you look at the middle of the remaining pages (from O to Z). If you land on “squirrel,” you know “penguin” comes before “squirrel,” so you can ignore the second half of these remaining pages.
- Keep halving the dictionary. Each time, you divide the remaining section in half, looking at the middle word and narrowing down your search until you land on “penguin.”
Splitting the search area in half with each step greatly reduces the number of pages you need to look through. This divide and conquer method makes binary search more efficient than checking every word sequentially.
Analogy Breakdown:
- Divide: You split the dictionary (problem) in half with each guess.
- Conquer: You only focus on the remaining half that could contain the word (solution).
- Recursive nature: The same process is repeated on the smaller and smaller dictionary sections until the word is found.
Why Binary Search is Efficient
The main strength of Binary Search lies in its logarithmic time complexity, O(log n). As the input size grows, Binary Search becomes far more efficient than a linear search. For example, searching for an element in a list of 1,000,000 elements requires at most 20 comparisons, compared to 1,000,000 comparisons in a linear search.
This makes Binary Search a go-to choice for scenarios where:
- The dataset is large and sorted.
- Quick lookup times are essential.
- Memory usage is a concern, as Binary Search doesn’t require extra space beyond the input data.
Pre-requisites for Binary Search
Binary Search does have a few important requirements:
- The collection must be sorted: Binary Search only works on a sorted array or list. If the data is unsorted, you must sort it first (typically O(n log n) before applying Binary Search.
- Data structure support: While Binary Search is usually implemented on arrays or lists, it can also be used on other sorted data structures like binary search trees or in applications like databases and file systems.
Binary Search Pseudocode
Now that we understand the theory, let’s examine the pseudocode for Binary Search. Below are two common ways to implement Binary Search: the iterative and recursive approaches.
Iterative Approach
Iterative means that the algorithm uses a loop to repeatedly adjust the search boundaries until the target is found or the search space is exhausted. It keeps narrowing down the search space by adjusting left
and right
pointers inside a while
loop, which makes it simple and efficient.
function binarySearch(arr, target): left = 0 right = length(arr) - 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid // Target found at index mid elif arr[mid] < target: left = mid + 1 // Search the right half else: right = mid - 1 // Search the left half return -1 // Target not found
Explanation:
In this version, we start with the whole array (left = 0
and right = length(arr) - 1
). Each iteration checks the middle element, and based on the comparison, the algorithm adjusts the search space by modifying left
or right
. The loop continues until either the target is found or left
exceeds right
.
Recursive Approach
Recursive means that the algorithm solves the problem by calling itself with a smaller portion of the input, reducing the problem size with each call. In this version of Binary Search, the function repeatedly calls itself with updated boundaries (left
and right
) until the target is found or the search space is empty.
function binarySearchRecursive(arr, left, right, target): if left > right: return -1 // Target not found mid = (left + right) // 2 if arr[mid] == target: return mid // Target found at index mid elif arr[mid] < target: return binarySearchRecursive(arr, mid + 1, right, target) // Search the right half else: return binarySearchRecursive(arr, left, mid - 1, target) // Search the left half
Explanation:
In the recursive version, the problem is broken down into smaller sub-problems. If the middle element is not the target, the algorithm calls itself recursively, either on the left or right half of the array. The recursion continues until the target is found or the base case (left > right
) is met, signalling that the target is absent in the array.
Binary Search Code Implementations
This section explains how to implement the algorithm in various programming languages. Binary Search can be written in two main ways: iterative and recursive.
- Iterative implementation: This approach uses a loop to perform the search, making it straightforward to track the search progress.
- Recursive implementation: This approach breaks the problem into smaller subproblems, using function calls to handle each new search space.
Iterative Implementations
def binary_search_iterative(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
arr = [1, 3, 5, 7, 9]
print(binary_search_iterative(arr, 5)) # Output: 2
#include <iostream>
#include <vector>
int binarySearchIterative(const std::vector<int>& arr, int target) {
int left = 0, right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
else if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
int main() {
std::vector<int> arr = {1, 3, 5, 7, 9};
std::cout << binarySearchIterative(arr, 5); // Output: 2
}
import java.util.List;
public class BinarySearchIterative {
public static int binarySearchIterative(List<Integer> arr, int target) {
int left = 0, right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr.get(mid) == target) return mid;
else if (arr.get(mid) < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
public static void main(String[] args) {
List<Integer> arr = List.of(1, 3, 5, 7, 9);
System.out.println(binarySearchIterative(arr, 5)); // Output: 2
}
}
function binarySearchIterative(arr, target) {
let left = 0, right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
else if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
const arr = [1, 3, 5, 7, 9];
console.log(binarySearchIterative(arr, 5)); // Output: 2
Recursive Implementations
def binary_search_recursive(arr, target, left, right):
if left > right:
return -1 # Base case: not found
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
return binary_search_recursive(arr, target, mid + 1, right)
else:
return binary_search_recursive(arr, target, left, mid - 1)
arr = [1, 3, 5, 7, 9]
print(binary_search_recursive(arr, 5, 0, len(arr) - 1)) # Output: 2
#include <iostream>
#include <vector>
int binarySearchRecursive(const std::vector<int>& arr, int target, int left, int right) {
if (left > right) return -1; // Base case: not found
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
else if (arr[mid] < target) return binarySearchRecursive(arr, target, mid + 1, right);
else return binarySearchRecursive(arr, target, left, mid - 1);
}
int main() {
std::vector<int> arr = {1, 3, 5, 7, 9};
std::cout << binarySearchRecursive(arr, 5, 0, arr.size() - 1); // Output: 2
}
import java.util.List;
public class BinarySearchRecursive {
public static int binarySearchRecursive(List<Integer> arr, int target, int left, int right) {
if (left > right) return -1; // Base case: not found
int mid = left + (right - left) / 2;
if (arr.get(mid) == target) return mid;
else if (arr.get(mid) < target) return binarySearchRecursive(arr, target, mid + 1, right);
else return binarySearchRecursive(arr, target, left, mid - 1);
}
public static void main(String[] args) {
List<Integer> arr = List.of(1, 3, 5, 7, 9);
System.out.println(binarySearchRecursive(arr, 5, 0, arr.size() - 1)); // Output: 2
}
}
function binarySearchRecursive(arr, target, left, right) {
if (left > right) return -1; // Base case: not found
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
else if (arr[mid] < target) return binarySearchRecursive(arr, target, mid + 1, right);
else return binarySearchRecursive(arr, target, left, mid - 1);
}
const arr = [1, 3, 5, 7, 9];
console.log(binarySearchRecursive(arr, 5, 0, arr.length - 1)); // Output: 2
Time and Space Complexity Considerations in Binary Search
When discussing binary search, it’s essential to consider not only its time complexity but also its space complexity, which depends on the implementation—iterative or recursive. While both implementations have the same time complexity, their space complexity differs due to how they handle function calls and memory allocation.
Time Complexity of Binary Search
Both iterative and recursive implementations of binary search have a time complexity of O(log n). This is because, with each step, the algorithm divides the search space in half, reducing the problem size by a factor of two. The number of steps required to reach a single element is proportional to the logarithm (base 2) of the input size.
- Iterative and Recursive: O(log n)
Space Complexity of Iterative Binary Search
In the iterative implementation of binary search, the algorithm uses a loop to halve the search space repeatedly. Since the iterative version does not involve additional memory allocation for function calls, its space complexity is constant—O(1). The only extra memory used is for a few variables, such as left, right, and mid, which stores indices in the array.
- Space Complexity: O(1)
The iterative version only uses a fixed amount of memory, regardless of the input array size.
Space Complexity of Recursive Binary Search
In the recursive implementation of binary search, the algorithm reduces the problem size by recursively calling itself on a smaller portion of the array. Each recursive call adds a new frame to the call stack, which consumes additional memory. The recursion depth depends on how often the problem is halved, which is O(log n) in the worst case.
Each recursive call stores the current state (local variables like left
, right
, and mid
), and these calls remain on the stack until the base case (when left > right
or the target is found) is reached. As a result, the space complexity of recursive binary search is O(log n), due to the additional stack frames.
- Space Complexity: O(log n)
The recursive version uses additional stack space proportional to the depth of the recursion, which is O(log n) in the worst case.
Practical Applications of Binary Search
Binary search is a foundational algorithm with broad applications across various fields in computer science and real-world problem-solving. Its efficiency makes it ideal for tasks that require searching large, sorted datasets. Below are some key practical applications.
Searching in Large Databases
In large, sorted databases, binary search is used to locate records based on specific keys quickly. For example, searching through a customer database sorted by names or IDs can be done in O(log n) time, drastically improving performance over linear search.
- Use Case: Find user profiles, orders, or records in sorted datasets quickly.
Indexing in Search Engines
Search engines use binary search to locate specific pages or keywords in their vast, indexed datasets. Binary search allows fast lookups of the relevant web pages for a search query by maintaining a sorted index of words or phrases.
- Use Case: Quickly retrieving documents or websites that contain a keyword.
Finding Boundaries in Ranges
Binary search is used to find specific boundaries, such as the first or last occurrence of a target in a sorted array (e.g., the range of elements that match a query in data). It is useful in interval queries or range-based algorithms.
- Use Case: Finding the start or end position of a target range in financial data or logs.
Efficiently Solving Optimization Problems
Binary search can solve optimization problems where you must find the optimal value that meets a condition, such as minimizing or maximizing a function. You can efficiently converge on the solution by applying binary search over a range of possible values.
- Use Case: Finding the best parameters for machine learning models or determining thresholds in data analysis.
Binary Search in Libraries and Functions
Many programming libraries, like Python’s bisect
module or Java’s Collections.binarySearch()
, implement binary search to handle sorted collections efficiently. These libraries allow developers to quickly search for elements in lists or arrays without implementing the algorithm from scratch.
- Use Case: Efficient searching in built-in data structures like lists, arrays, and sets.
Solving Mathematical Problems
Binary search is often applied in mathematical and algorithmic problems, such as finding square roots of numbers or approximating solutions to equations. In these cases, binary search can iterate over a range of values to find the correct solution with a precision specified by the user.
- Use Case: Finding square roots, roots of polynomials, or approximating real numbers.
Networking and Load Balancing
Binary search can be used in distributed systems to balance loads, allocate resources, or efficiently search through distributed datasets. For instance, distributed hash tables (DHTs) in peer-to-peer networks use binary search-like algorithms to locate data in a distributed fashion.
- Use Case: Load balancing in distributed computing or searching for data in peer-to-peer networks.
Binary Search on Different Data Structures
Binary search is commonly associated with sorted arrays, but its principles can be applied to other data structures. The underlying concept—dividing the search space in half—can be extended to binary search trees (BSTs) and strings with different nuances.
Arrays: The Traditional Use Case
In arrays, binary search directly accesses the middle element using its index. This is possible because arrays provide random access, allowing quick lookups in O(1) time. Binary search iteratively or recursively compares the target with the middle element and narrows down the search space by halving the array.
- Key Requirement: The array must be sorted. If the array is unsorted, the binary search will not work correctly.
- Time Complexity: O(log n)
- Space Complexity: O(1) for the iterative version, O(log n) for the recursive version.
Binary Search Trees (BSTs): A Natural Application
Binary search trees (BSTs) are structured to support binary search naturally. In a BST:
- The left subtree contains values smaller than the root.
- The right subtree contains values larger than the root.
Binary search starts at the root and recursively moves left or right based on comparisons, like halving the search space in an array. Since the tree is ordered, each traversal eliminates half of the remaining nodes.
- Key Requirement: The tree must be balanced for optimal performance. In unbalanced trees, the search can degrade to O(n).
- Time Complexity: O(log n) in balanced trees; O(n) in unbalanced trees.
Strings: Searching for Substrings or Words
Binary search can also be applied to a sorted list of strings. Comparisons between strings are done lexicographically (in alphabetical order). Like in arrays, binary search compares the target string with the middle string and narrows the search space by halving the list.
- Key Requirement: The list of strings must be sorted lexicographically.
- Time Complexity: O(log n) for searching in a sorted list of strings.
Example Use Cases:
- Searching for a specific word in a dictionary or a sorted list of words.
- Locating a particular substring or target string in large sorted datasets, such as logs or documents.
Edge Cases and Pitfalls in Binary Search
Although binary search is a highly efficient algorithm, it’s important to know certain edge cases and common pitfalls that can arise during implementation. These can cause incorrect results, infinite loops, or performance issues if not handled properly. Below are some critical edge cases, pitfalls you should watch out for, and strategies to avoid them.
Handling Missing Elements
Binary search assumes that the element you are searching for may not always be present in the array. If not handled correctly, this can lead to misleading results, such as returning incorrect indices or causing the algorithm to loop indefinitely.
Example:
Suppose you’re searching for a value x
in a sorted array arr
. If the value is not in the array, your search will eventually narrow down to an empty search space, where left
becomes greater than right
. If this edge case isn’t explicitly handled, the algorithm could behave unpredictably or crash.
Solution:
Always check if the left
pointer exceeds the right
pointer to terminate the search. If the value isn’t found, return a special value, such as -1
or a clear indication that the element is not present.
if left > right: return -1 # Indicating that the element is not found
Infinite Loops Due to Incorrect Pointer Updates
A very common pitfall in binary search is the improper update of the left
or right
pointers, which can lead to an infinite loop. This happens if the middle element is not correctly excluded from subsequent searches.
Example:
If the midpoint is calculated as:
mid = (left + right) // 2
and the condition is:
if arr[mid] == target: return mid elif arr[mid] < target: left = mid # This is incorrect! else: right = mid # This is also incorrect!
If left
is set to mid
(or right
to mid
), and mid
remains the same on subsequent iterations, the search can get stuck in an infinite loop.
Solution:
Always ensure that the middle element is excluded from further searches by using left = mid + 1
or right = mid - 1
, depending on the comparison. This guarantees that the search space will be reduced with each iteration.
if arr[mid] < target: left = mid + 1 # Move past the middle element else: right = mid - 1 # Move before the middle element
Integer Overflow in Midpoint Calculation
In languages like C++ or Java, using large arrays can cause an integer overflow when calculating the middle index, especially when the array’s size approaches the maximum value of an integer.
Example:
In systems where integers have a fixed size (e.g., 32 bits), calculating the midpoint as mid = (left + right) / 2
can lead to overflow if left
and right
are very large, causing incorrect behaviour.
Solution:
To avoid overflow, a safer way to calculate the midpoint is:
mid = left + (right - left) // 2
This avoids adding left
and right
directly and ensures that the calculation doesn’t overflow, even with large values.
Searching in a Rotated Sorted Array
A rotated sorted array is one that was initially sorted but then “rotated” (shifted) by some number of positions. Binary search won’t work out of the box for rotated arrays because the order is no longer ascending or descending across the entire array.
Example:
Consider the array [6, 7, 8, 1, 2, 3, 4, 5]
, which is a rotated version of [1, 2, 3, 4, 5, 6, 7, 8]
. A regular binary search will fail if the array isn’t globally sorted.
Solution:
To handle a rotated sorted array, modify the binary search logic to account for the rotation. First, determine which half of the array is sorted, and then apply binary search on the relevant half.
if arr[left] <= arr[mid]: # Left half is sorted if target >= arr[left] and target <= arr[mid]: right = mid - 1 else: left = mid + 1 else: # Right half is sorted if target >= arr[mid] and target <= arr[right]: left = mid + 1 else: right = mid - 1
Off-by-One Errors
Off-by-one errors can occur when managing index boundaries (left
and right
). Incorrectly setting these boundaries can result in missing elements that should be found or unnecessary infinite loops.
Example:
This often happens when handling inclusive/exclusive ranges. If you mistakenly write:
left = mid # Should be mid + 1
or
right = mid # Should be mid - 1
You may accidentally recheck the same middle element indefinitely, leading to an infinite loop.
Solution:
Be cautious with boundary conditions. Use inclusive or exclusive ranges consistently and remember to adjust left
or right
correctly after comparisons. For example:
if arr[mid] < target: left = mid + 1 # Move to the right half else: right = mid - 1 # Move to the left half
Duplicates in the Array
When the array contains duplicate values, binary search might not find the first or last occurrence of the target. By default, binary search returns any occurrence, which may not always be the first or last one.
Example:
If the array is [1, 2, 2, 2, 3, 4]
and you’re searching for 2
, the binary search might return the index of any of the 2
s, but not necessarily the first one.
Solution:
To find the first or last occurrence of the target, modify the algorithm to continue searching even after finding the target. For example, to find the first occurrence:
if arr[mid] == target: result = mid right = mid - 1 # Continue searching to the left for earlier occurrences
For the last occurrence, continue searching to the right:
if arr[mid] == target: result = mid left = mid + 1 # Continue searching to the right for later occurrences
Incorrect Handling of Edge Indices
It’s easy to overlook edge conditions at the very start or end of the array, where the search might fail or behave incorrectly.
Example:
In an array with one or two elements, it’s easy to mismanage the boundary conditions and end up with left
and right
out of sync.
Solution:
Ensure that the algorithm properly handles arrays of all sizes, including edge cases like an array of length 1, and avoids accessing out-of-bounds indices.
Binary Search: When It’s Not the Best Choice
Binary search is an efficient algorithm for finding elements in a sorted dataset with a time complexity of O(log n)
. However, one key limitation is that the data must be sorted. If the dataset is unsorted, the cost of sorting it first (O(n log n)
) can outweigh the benefits of binary search. A linear search may be more practical in such cases, especially for smaller or unsorted datasets, as it avoids the sorting overhead.
Data Structure Considerations
Binary search relies on random access to data, which is why it works best with data structures like arrays, where elements can be accessed directly by their index in constant time. It is inefficient for linked lists, where accessing the middle element requires sequential traversal, making binary search impractical. Maintaining sorted order in an array can be expensive for dynamic datasets that require frequent insertions and deletions. In such cases, balanced binary search trees or hash tables are better alternatives, as they support efficient searching and frequent updates.
When Searching for Ranges
Binary search is less suitable for problems that involve searching for ranges or intervals of elements, as it is optimized for finding a single element. Specialized data structures like segment trees or interval trees provide better performance in these cases. While binary search is a powerful tool, it’s important to consider the specific characteristics of the problem and data structure before deciding to use it.
Optimizations and Variations of Binary Search
While binary search is already an efficient algorithm, several optimizations and variations can be applied to adapt it to different scenarios. Below are some key approaches that enhance or extend the basic binary search.
Exponential Search
Exponential search is an optimization that combines binary search with an initial probing step. It’s beneficial for unbounded or very large arrays where the size is unknown or infinite. The algorithm starts by exponentially increasing an index to find the target range and then applies binary search within that range.
- Use Case: Searching in unbounded arrays or arrays where the upper limit is unknown.
- Time Complexity: O(log n), where n is the position of the target element.
Ternary Search
Ternary search is a variation of binary search where the array is divided into three parts instead of two. It’s useful when searching for the maximum or minimum value in a unimodal function (a function that increases and then decreases).
- Use Case: Finding the extremum (maximum or minimum) in a unimodal array.
- Time Complexity: O(log n), similar to binary search, but it can sometimes be less efficient in practice due to the added complexity of splitting the array into three parts.
Interpolation Search
Interpolation search is an improvement over binary search for uniformly distributed arrays. Instead of always choosing the middle element, it estimates the position of the target based on its value relative to the range of the data. This technique reduces the number of comparisons in uniformly distributed data.
- Use Case: Searching in large arrays with uniformly distributed values.
- Time Complexity: O(log log n) in the best case, but O(n) in the worst case (for non-uniform distributions).
Binary Search Variants for Duplicates
Binary search can be modified to handle cases where the array contains duplicate elements. Two common variants are:
- First Occurrence: Find the first occurrence of the target by continuing the search to the left even after the target is found.
- Last Occurrence: Find the last occurrence by searching to the right.
These variants are helpful when searching for a target that may appear multiple times in the array.
- Use Case: Finding the first or last occurrence of a repeated element.
- Time Complexity: O(log n).
Search for Range Boundaries
Binary search can also be extended to search for range boundaries, such as the smallest element greater than or equal to a given target or the largest element smaller than or equal to a target. This is particularly useful in range queries or finding elements that meet a condition.
- Use Case: Solving problems where finding boundary elements is crucial, like searching for the smallest/largest element meeting a condition.
- Time Complexity: O(log n).
Binary Search in Rotated Sorted Arrays
When working with a rotated sorted array, such as [6, 7, 1, 2, 3, 4, 5]
, binary search must account for the rotation. A modified version of binary search can first determine which half of the array is sorted and then search in the relevant half.
- Use Case: Searching in rotated arrays that are still partially sorted.
- Time Complexity: O(log n).
Conclusion
Binary Search is an indispensable algorithm in computer science, prized for its efficiency and simplicity. Its logarithmic time complexity allows it to search large sorted datasets quickly, making it far more efficient than linear search. While primarily used with arrays, binary search is versatile enough to be applied to other data structures, such as binary search trees and sorted lists of strings, adapting well to various scenarios. We explored how the iterative version is memory efficient, while the recursive version aligns with divide-and-conquer principles but requires additional stack space. Furthermore, binary search can be optimized for unbounded arrays, uniform distributions, and even rotated datasets, showing its flexibility.
In practical terms, binary search is widely used in databases, search engines, libraries, and optimization problems, proving its relevance across numerous fields. Whether you’re finding boundaries, solving mathematical problems, or implementing it in distributed systems, mastering binary search is essential for any programmer. Its role as a foundational algorithm for more advanced techniques and data structures solidifies its importance, making it a core tool that every engineer should understand and utilize effectively.
Congratulations on reading to the end of this tutorial!
For further reading on using binary search for inserting elements into sorted datasets, read the article Binary Search Insertion: A Comprehensive Guide
For further reading on binary search in specific languages:
For more information on data structures and algorithms, visit our DSA page!
Have fun and happy researching!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.