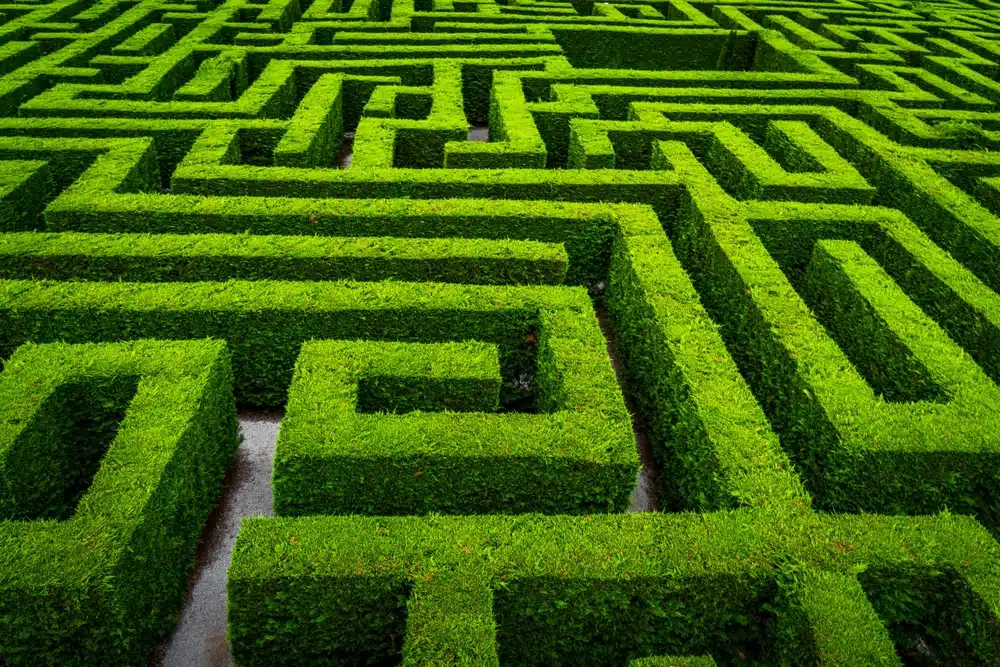
Finding the shortest path in a graph is a fundamental problem in computer science, with applications in navigation, networking, and game AI. This guide breaks down Dijkstra’s algorithm step by step, from theory to implementation.
Table of Contents
Introduction
Dijkstra's algorithm stands as one of the most fundamental and elegant solutions in computer science for finding the shortest paths between nodes in a weighted graph. Developed by Edsger W. Dijkstra in 1956 and published in 1959, this algorithm has become the cornerstone of pathfinding applications, from network routing protocols to GPS navigation systems.
Key Features
- Single-Source: Finds shortest paths from a single source node to all other nodes
- Non-negative Weights: Works with graphs where all edge weights are non-negative
- Greedy Strategy: Uses a greedy approach to systematically select the minimum-distance vertex
- Optimality: Guarantees the shortest path in weighted directed graphs with non-negative edges
Prerequisites
To fully understand Dijkstra's algorithm, you should be familiar with:
- Graph Theory Basics: Vertices, edges, weighted graphs, and directed vs undirected graphs
- Data Structures: Priority queues, adjacency lists/matrices, and basic heap operations
- Algorithm Concepts: Greedy algorithms and the principle of relaxation
Important Considerations
While Dijkstra's algorithm is powerful, it comes with certain limitations:
- Cannot handle negative edge weights; use Bellman-Ford for graphs with negative weights.
- Dijkstra’s is efficient for sparse graphs but can be slow for dense graphs.
- Explores nodes in all directions, which may be inefficient when a single destination is needed; A* can be more efficient in such cases.
Core Concept
At its heart, Dijkstra's algorithm maintains a set of unvisited nodes and continuously updates the shortest distance to each node. It follows a simple but powerful principle:
The Principle of Relaxation
If we have found a path to vertex v, and there is an edge from v to w, then the path to w through v might be better than the currently known path to w. We should thus "relax" the current distance to w if the path through v is shorter.
# High-level view of Dijkstra's algorithm
1. Initialize distances (infinity for all vertices except source = 0)
2. While there are unvisited nodes:
a. Select unvisited node with minimum distance
b. Mark it as visited
c. Update distances to its unvisited neighbors through relaxation
Interactive Visualization
Node | A | B | C | D | E | F |
---|---|---|---|---|---|---|
Distance | 0 | ∞ | ∞ | ∞ | ∞ |
Time and Space Complexity
- Time Complexity: O((V + E) log V) using a binary heap, where each vertex is dequeued once, and edges are relaxed at most once.
- Space Complexity: O(V) for the distance array and priority queue, plus O(E) for adjacency lists (if used).
- Optimized Variants: Can achieve O(E + V log V) using Fibonacci heaps, which improve decrease-key operations.
In the following sections, we'll dive deep into the mathematical foundation of the algorithm, explore various implementations in popular programming languages, and compare it with other pathfinding algorithms. Whether you're implementing a navigation system, network router, or solving graph problems in competitive programming, understanding Dijkstra's algorithm is essential.
Try it Yourself: Interactive Pathfinding Visualizer
Experiment with Dijkstra's algorithm in action! Generate random mazes, visualize the pathfinding process, and compare different algorithms including Dijkstra's, A*, Bellman-Ford, BFS, and DFS. Features real-time animations and path-related statistics.
🧠 Pathfinding Showdown: Algorithm Comparison
Watch five powerful algorithms race through intricate mazes in this interactive visualization that showcases their unique search patterns and efficiency with beautiful animations and real-time metrics. Compare how Dijkstra's methodical approach differs from A*'s heuristic-guided search, BFS's level-by-level exploration, DFS's deep diving technique, and Bellman-Ford's ability to handle complex weight scenarios.
The visualization demonstrates real-time statistics including nodes visited, path length, execution time, and memory usage, allowing you to directly observe the efficiency tradeoffs between these algorithms in action.
Mathematical Background
Formal Definition
Given a weighted graph \(G = (V, E)\), where:
- \(V = \{A, B, C, D, E, F\}\) is the set of vertices
- \(E \subset V \times V\) is the set of edges
- \(w: E \to \mathbb{R}_{\geq 0}\) is the weight function
- \(d: V \to \mathbb{R}_{\geq 0} \cup \{\infty\}\) is the distance function where: \[ d(v) = \begin{cases} 0 & \text{if } v = s \\ \infty & \text{otherwise} \end{cases} \]
- \(s \in V\) is the source vertex (in our case, \(s = A\))
At each iteration, the algorithm maintains two disjoint sets:
\[ S = \{ v \in V \mid d(v) \text{ is finalized}\} \] \[ Q = V \setminus S = \{ v \in V \mid d(v) \text{ may be improved}\} \]where \( Q \) contains vertices for which the shortest path distance has not yet been finalized and may still be updated.
With the invariant property that for all \(v \in S\):
\[ d(v) = \delta(s,v) \] where \( \delta(s,v) \) is the function that gives the shortest path distance from \( s \) to \( v \) in \( G \).The relaxation operation for an edge \((u,v) \in E\) is defined as:
\[ d(v) \leftarrow \min\{d(v), d(u) + w(u,v)\} \]If \( d(v) > d(u) + w(u,v) \), update \( d(v) \) and set \( u \) as its predecessor.
ELI5: Understanding the Formal Definition
Think of a city map where each location is a vertex and roads between them are edges. Each road has a weight, like the time or distance to travel it.
- Goal: Find the shortest travel route from one starting point (source) to all other locations.
- Distance Array: Imagine a list where we write down the shortest known travel time to each location.
- Visited Set: These are places we've fully explored, meaning we know the best way to reach them.
- Unvisited Set: These are locations we haven’t finalized yet; we might still find a shorter route.
- Relaxation: Every time we find a new path, we check if it's shorter than what we previously recorded. If it is, we update our notes.
By repeating this process, we eventually lock in the shortest paths to all locations, just like a GPS does when planning the fastest route!
Graph Structure
Our example graph has the following adjacency matrix representation:
A | B | C | D | E | F | |
---|---|---|---|---|---|---|
A | 0 | 4 | 2 | ∞ | ∞ | ∞ |
B | 4 | 0 | ∞ | 3 | ∞ | ∞ |
C | 2 | ∞ | 0 | ∞ | 1 | ∞ |
D | ∞ | 3 | ∞ | 0 | 4 | 4 |
E | ∞ | ∞ | 1 | 4 | 0 | 3 |
F | ∞ | ∞ | ∞ | 4 | 3 | 0 |
Highlighted cells show the edges in the shortest path from A to F
Path Analysis
Our graph offers these paths from A to F:
- A → C → E → F (total: 2 + 1 + 3 = 6) - Optimal Path
- A → B → D → F (total: 4 + 3 + 4 = 11)
- A → C → E → D → F (total: 2 + 1 + 4 + 4 = 11)
Correctness Proof
The correctness of Dijkstra’s algorithm relies on two key properties:
1. Optimal Substructure
Let \( p \) be a shortest path from \( s \) to \( v \). Then any subpath of \( p \) is also a shortest path between its endpoints.
Proof by Contradiction: If a subpath \( p' \) of \( p \) were not the shortest, we could replace it with a shorter path, contradicting the optimality of \( p \).
2. Greedy Choice Property
Dijkstra's algorithm always expands the vertex with the smallest known shortest distance, ensuring that once a vertex is processed, its shortest distance is finalized.
Justification: Because all edge weights are non-negative, once a vertex \( v \) is dequeued from the priority queue, there is no shorter path to \( v \) that remains undiscovered.
Key Properties Demonstrated
- Greedy Choice Property:
At each step, selecting the vertex with minimum \(d[v]\) leads to the optimal solution. In our example, choosing C (distance 2) over B (distance 4) leads to the optimal path.
- Path Construction:
The final path A→C→E→F is constructed by following the sequence of optimal choices:
- A→C (weight 2): First edge on shortest path
- C→E (weight 1): Second edge, maintaining optimality
- E→F (weight 3): Final edge completing shortest path
- Alternative paths through B→D→F and E→D→F (both total 11) are considered but rejected
- Exhaustive Verification:
The algorithm explores all potential paths, including:
- Direct paths from discovered vertices to F
- Indirect paths through intermediate vertices
- Alternative paths even after finding a potential solution
Implementation
We'll explore implementations of Dijkstra's algorithm in multiple programming languages. Each implementation includes both a standard version and an optimized version using a priority queue for better performance.
Implementation Strategy
- Use priority queue for efficient minimum distance selection
- Support both adjacency matrix and adjacency list representations
- Include path reconstruction
- Provide comprehensive error handling
Python Implementation
Uses Python's heapq module for the priority queue implementation and includes comprehensive path tracking.
from typing import Dict, List, Set, Tuple, Optional
import heapq
class Graph:
def __init__(self, vertices: int): # Fixed double underscore syntax
"""Initialize the graph with given number of vertices."""
self.V = vertices
self.graph = [[0 for _ in range(vertices)] for _ in range(vertices)] # Fixed placeholder variable
def add_edge(self, u: int, v: int, weight: int) -> None:
"""Add an edge to the graph."""
self.graph[u][v] = weight
self.graph[v][u] = weight # For undirected graph
class DijkstraAlgorithm:
def __init__(self, graph: Graph): # Fixed double underscore syntax
"""Initialize Dijkstra's algorithm with a graph."""
self.graph = graph
self.V = graph.V
def find_shortest_path(self, src: int, dest: int) -> Tuple[List[int], int]:
"""
Find shortest path between src and dest vertices.
Returns tuple of (path, distance)
"""
distances = [float('inf')] * self.V
distances[src] = 0
pq = [(0, src)] # (distance, vertex)
previous = [-1] * self.V
visited = set()
while pq:
current_distance, current_vertex = heapq.heappop(pq)
if current_vertex in visited:
continue
visited.add(current_vertex)
if current_vertex == dest:
path = self._reconstruct_path(previous, src, dest) # Fixed method name
return path, distances[dest]
for neighbor in range(self.V):
if self.graph.graph[current_vertex][neighbor] > 0:
distance = (current_distance +
self.graph.graph[current_vertex][neighbor])
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_vertex
heapq.heappush(pq, (distance, neighbor))
return [], float('inf')
def _reconstruct_path(self, previous: List[int], src: int, dest: int) -> List[int]: # Fixed method name
"""Reconstruct path from source to destination."""
path = []
current = dest
while current != -1:
path.append(current)
current = previous[current]
path = path[::-1]
return path if path[0] == src else []
def main():
graph = Graph(6) # Vertices A(0) through F(5)
edges = [
(0, 1, 4), # A-B
(0, 2, 2), # A-C
(1, 3, 3), # B-D
(2, 4, 1), # C-E
(3, 5, 4), # D-F
(4, 5, 3), # E-F
(3, 4, 4), # D-E
]
for u, v, w in edges:
graph.add_edge(u, v, w)
dijkstra = DijkstraAlgorithm(graph)
path, distance = dijkstra.find_shortest_path(0, 5)
vertex_labels = {0: 'A', 1: 'B', 2: 'C', 3: 'D', 4: 'E', 5: 'F'}
path_letters = [vertex_labels[v] for v in path]
print(f"Shortest path: {' → '.join(path_letters)}")
print(f"Total distance: {distance}")
if __name__ == "__main__":
main()
Output:
Shortest path: A → C → E → F Total distance: 6
Java Implementation
Uses a PriorityQueue for efficient minimum vertex selection and includes path tracking.
import java.util.*;
public class DijkstraAlgorithm {
private final int V;
private final List<List<Edge>> graph;
// Edge class to represent weighted edges
private static class Edge {
int destination;
int weight;
Edge(int destination, int weight) {
this.destination = destination;
this.weight = weight;
}
}
// Node class for priority queue
private static class Node implements Comparable<Node> {
int vertex;
int distance;
Node(int vertex, int distance) {
this.vertex = vertex;
this.distance = distance;
}
@Override
public int compareTo(Node other) {
return Integer.compare(this.distance, other.distance);
}
}
public DijkstraAlgorithm(int vertices) {
this.V = vertices;
this.graph = new ArrayList<>(vertices);
for (int i = 0; i < vertices; i++) {
this.graph.add(new ArrayList<>());
}
}
public void addEdge(int source, int destination, int weight) {
graph.get(source).add(new Edge(destination, weight));
graph.get(destination).add(new Edge(source, weight)); // For undirected graph
}
public ShortestPathResult findShortestPath(int source, int destination) {
// Initialize distances and previous vertices
int[] distances = new int[V];
int[] previous = new int[V];
Arrays.fill(distances, Integer.MAX_VALUE);
Arrays.fill(previous, -1);
distances[source] = 0;
// Priority queue for vertex selection
PriorityQueue<Node> pq = new PriorityQueue<>();
pq.offer(new Node(source, 0));
// Set to track visited vertices
Set<Integer> visited = new HashSet<>();
while (!pq.isEmpty()) {
Node current = pq.poll();
if (visited.contains(current.vertex)) {
continue;
}
visited.add(current.vertex);
// If we reached destination, construct path and return
if (current.vertex == destination) {
return new ShortestPathResult(
reconstructPath(previous, source, destination),
distances[destination]
);
}
// Check all neighbors
for (Edge edge : graph.get(current.vertex)) {
if (!visited.contains(edge.destination)) {
int newDistance = distances[current.vertex] + edge.weight;
if (newDistance < distances[edge.destination]) {
distances[edge.destination] = newDistance;
previous[edge.destination] = current.vertex;
pq.offer(new Node(edge.destination, newDistance));
}
}
}
}
// No path found
return new ShortestPathResult(new ArrayList<>(), Integer.MAX_VALUE);
}
private List<Integer> reconstructPath(int[] previous,
int source, int destination) {
List<Integer> path = new ArrayList<>();
int current = destination;
while (current != -1) {
path.add(current);
current = previous[current];
}
Collections.reverse(path);
return path.get(0) == source ? path : new ArrayList<>();
}
// Class to hold result of shortest path search
public static class ShortestPathResult {
public final List<Integer> path;
public final int distance;
public ShortestPathResult(List<Integer> path, int distance) {
this.path = path;
this.distance = distance;
}
}
public static void main(String[] args) {
// Create graph from visualization example
DijkstraAlgorithm graph = new DijkstraAlgorithm(6); // A-F
// Add edges
int[][] edges = {
{0, 1, 4}, // A-B
{0, 2, 2}, // A-C
{1, 3, 3}, // B-D
{2, 4, 1}, // C-E
{3, 5, 4}, // D-F
{4, 5, 3}, // E-F
{3, 4, 4} // D-E
};
for (int[] edge : edges) {
graph.addEdge(edge[0], edge[1], edge[2]);
}
// Find shortest path from A(0) to F(5)
ShortestPathResult result = graph.findShortestPath(0, 5);
// Convert numeric vertices to letters for output
char[] vertexLabels = {'A', 'B', 'C', 'D', 'E', 'F'};
String path = result.path.stream()
.map(v -> String.valueOf(vertexLabels[v]))
.reduce((a, b) -> a + " → " + b)
.orElse("");
System.out.println("Shortest path: " + path);
System.out.println("Total distance: " + result.distance);
}
}
Output:
Shortest path: A → C → E → F Total distance: 6
JavaScript Implementation
Uses a custom PriorityQueue implementation since JavaScript doesn't have a built-in one.
class PriorityQueue {
constructor() {
this.values = [];
}
enqueue(vertex, priority) {
this.values.push({ vertex, priority });
this.sort();
}
dequeue() {
return this.values.shift();
}
sort() {
this.values.sort((a, b) => a.priority - b.priority);
}
}
class Graph {
constructor() {
this.adjacencyList = new Map();
}
addVertex(vertex) {
if (!this.adjacencyList.has(vertex)) {
this.adjacencyList.set(vertex, []);
}
}
addEdge(vertex1, vertex2, weight) {
this.adjacencyList.get(vertex1).push({ node: vertex2, weight });
this.adjacencyList.get(vertex2).push({ node: vertex1, weight });
}
findShortestPath(start, finish) {
const nodes = new PriorityQueue();
const distances = new Map();
const previous = new Map();
const path = [];
let smallest;
for (let vertex of this.adjacencyList.keys()) {
if (vertex === start) {
distances.set(vertex, 0);
nodes.enqueue(vertex, 0);
} else {
distances.set(vertex, Infinity);
nodes.enqueue(vertex, Infinity);
}
previous.set(vertex, null);
}
while (nodes.values.length) {
smallest = nodes.dequeue().vertex;
if (smallest === finish) {
while (previous.get(smallest)) {
path.push(smallest);
smallest = previous.get(smallest);
}
path.push(start);
break;
}
if (smallest || distances.get(smallest) !== Infinity) {
for (let neighbor of this.adjacencyList.get(smallest)) {
let candidate = distances.get(smallest) + neighbor.weight;
if (candidate < distances.get(neighbor.node)) {
distances.set(neighbor.node, candidate);
previous.set(neighbor.node, smallest);
nodes.enqueue(neighbor.node, candidate);
}
}
}
}
return {
path: path.reverse(),
distance: distances.get(finish)
};
}
}
// Create the graph
const graph = new Graph();
// Add vertices
['A', 'B', 'C', 'D', 'E', 'F'].forEach(vertex => {
graph.addVertex(vertex);
});
// Add edges
const edges = [
['A', 'B', 4],
['A', 'C', 2],
['B', 'D', 3],
['C', 'E', 1],
['D', 'F', 4],
['E', 'F', 3],
['D', 'E', 4]
];
edges.forEach(([v1, v2, weight]) => {
graph.addEdge(v1, v2, weight);
});
// Find shortest path from A to F
const result = graph.findShortestPath('A', 'F');
console.log(`Shortest path: ${result.path.join(' → ')}`);
console.log(`Total distance: ${result.distance}`);
Output:
Shortest path: A → C → E → F Total distance: 6
C++ Implementation
Uses STL priority_queue for efficient implementation and includes path reconstruction.
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
#include <map>
using namespace std;
class Graph {
int V; // number of vertices
vector<vector<pair<int, int>>> adj; // adjacency list of {vertex, weight}
public:
explicit Graph(int vertices) : V(vertices) {
adj.resize(vertices);
}
void addEdge(int u, int v, int weight) {
adj[u].emplace_back(v, weight);
adj[v].emplace_back(u, weight); // For undirected graph
}
[[nodiscard]] pair<vector<int>, int> shortestPath(int src, int dest) const {
// Priority queue of {distance, vertex}
priority_queue<pair<int, int>,
vector<pair<int, int>>,
greater<>> pq;
// Initialize distances
vector<int> distances(V, numeric_limits<int>::max());
vector<int> previous(V, -1);
vector<bool> visited(V, false);
distances[src] = 0;
pq.emplace(0, src);
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
if (visited[u]) continue;
visited[u] = true;
if (u == dest) {
return {reconstructPath(previous, src, dest), distances[dest]};
}
// Check all adjacent vertices
for (const auto& edge : adj[u]) {
int v = edge.first;
int weight = edge.second;
if (!visited[v]) {
int newDist = distances[u] + weight;
if (newDist < distances[v]) {
distances[v] = newDist;
previous[v] = u;
pq.emplace(newDist, v);
}
}
}
}
return {vector<int>(), numeric_limits<int>::max()};
}
private:
static vector<int> reconstructPath(const vector<int>& previous,
int src, int dest) {
vector<int> path;
for (int current = dest; current != -1; current = previous[current]) {
path.push_back(current);
}
ranges::reverse(path);
return path[0] == src ? path : vector<int>();
}
};
int main() {
// Create graph from visualization example
Graph g(6); // Vertices 0(A) through 5(F)
// Add edges
vector<tuple<int, int, int>> edges = {
{0, 1, 4}, // A-B
{0, 2, 2}, // A-C
{1, 3, 3}, // B-D
{2, 4, 1}, // C-E
{3, 5, 4}, // D-F
{4, 5, 3}, // E-F
{3, 4, 4} // D-E
};
for (const auto& [u, v, w] : edges) {
g.addEdge(u, v, w);
}
// Find shortest path from A(0) to F(5)
auto [path, distance] = g.shortestPath(0, 5);
// Convert numeric vertices to letters for output
map<int, char> vertexLabels = {
{0, 'A'}, {1, 'B'}, {2, 'C'},
{3, 'D'}, {4, 'E'}, {5, 'F'}
};
cout << "Shortest path: ";
for (size_t i = 0; i < path.size(); ++i) {
if (i > 0) cout << " → ";
cout << vertexLabels[path[i]];
}
cout << "\nTotal distance: " << distance << endl;
return 0;
}
Output:
Shortest path: A → C → E → F Total distance: 6
Implementation Benefits
- Efficiency: Priority queue implementations provide O((V + E)log V) time complexity
- Flexibility: Works with both adjacency matrix and list representations
- Maintainability: Clean separation of graph and algorithm logic
- Reusability: Path reconstruction functionality can be used for other path-finding needs
Language-Specific Notes
- Python: Uses heapq for simple priority queue implementation
- Java: Built-in PriorityQueue provides efficient implementation
- JavaScript: Custom PriorityQueue class needed due to lack of built-in implementation
- C++: STL priority_queue offers best performance
Relationship to Other Path-Finding Algorithms
Dijkstra's algorithm is one of several fundamental algorithms for finding shortest paths in graphs. Understanding its relationship to other algorithms helps in choosing the right approach for specific use cases.
Algorithm | Relationship to Dijkstra's | Key Differences | Best Use Cases |
---|---|---|---|
Bellman-Ford |
|
|
|
Floyd-Warshall |
|
|
|
A* Search |
|
|
|
Feature Comparison Matrix
When selecting a shortest path algorithm, it’s crucial to consider factors like graph properties, computational complexity, memory usage, and optimality guarantees. Some algorithms excel in specific scenarios, such as handling negative weights, heuristic-driven pathfinding, or dense graph structures, while others are more efficient for real-time applications.
The following Feature Comparison Matrix provides a structured overview of how Dijkstra’s, Bellman-Ford, Floyd-Warshall, and A* differ across key characteristics. This will help you quickly identify the most suitable algorithm based on your specific requirements.
Feature | Dijkstra's | Bellman-Ford | Floyd-Warshall | A* |
---|---|---|---|---|
Negative Edges | ✗ | ✓ | ✓ | ✗ |
All Pairs | ✗ | ✗ | ✓ | ✗ |
Heuristic-guided | ✗ | ✗ | ✗ | ✓ |
Cycle Detection | ✗ | ✓ | ✓ (detects negative cycles) | ✗ |
Time Complexity | O((V + E) log V), O(V²) in dense graphs | O(VE) | O(V³) | O((V + E) log V)* |
Space Complexity | O(V) | O(V) | O(V²) | O(V) to O(E), depends on heuristic |
Optimality | ✓ | ✓ | ✓ | ✓ (only if heuristic is admissible and consistent)* |
* A* complexity depends on heuristic; given for an admissible heuristic.
* A* optimality is guaranteed only if the heuristic is admissible and consistent.
* Floyd-Warshall detects negative cycles by checking diagonal elements of the distance matrix.
* Space complexities shown are for basic implementations.
* Time complexities are worst-case scenarios.
Selection Guidelines
Consider these factors when choosing between algorithms:
- Graph Properties:
- Presence of negative weights → Bellman-Ford
- Dense graphs with multiple queries → Floyd-Warshall
- Non-negative weights for shortest paths → Dijkstra’s
- Spatial/Geographic routing → A*
- Performance Requirements:
- Limited memory → Bellman-Ford (no priority queue) or basic Dijkstra’s (array-based)
- Multiple path queries → Floyd-Warshall
- Real-time performance → A*
- Implementation Complexity:
- Simple but inefficient for large graphs → Floyd-Warshall
- No priority queue needed → Bellman-Ford
- Domain knowledge available → A*
Key Insights
- Performance vs. Flexibility: Dijkstra’s is efficient for non-negative weights, but Bellman-Ford is more flexible for graphs with negative weights.
- Memory Tradeoffs: Floyd-Warshall precomputes all-pairs shortest paths, trading memory for fast queries.
- Domain Knowledge: A* uses heuristics to leverage domain-specific knowledge for faster pathfinding.
- Optimality: These algorithms guarantee optimal solutions if their assumptions hold (e.g., no negative cycles for Dijkstra's/Bellman-Ford).
Conclusion
Throughout this guide, we've explored Dijkstra's algorithm - from its mathematical foundations to practical implementations and comparisons with other pathfinding algorithms. Its elegant combination of greedy strategy and optimal substructure makes it a powerful tool for solving shortest path problems with non-negative edge weights.
When to Use Dijkstra's Algorithm
- Non-negative edge weights are guaranteed
- Single-source shortest paths are needed
- Good trade-off between ease of implementation and efficiency in practical use cases
- Memory-efficient for single-source queries but not ideal for all-pairs shortest paths
Implementation Considerations
- Use a min-heap (binary heap or Fibonacci heap) for optimal performance in sparse graphs
- Adjacency lists (O(V log V + E)) for sparse graphs, adjacency matrices (O(V²)) for dense graphs
- Consider space-optimized versions for large-scale applications
- Always validate input graphs for non-negative weights
If you found this guide helpful, please consider citing or sharing it with fellow developers and algorithm enthusiasts. For more resources on graph algorithms, implementation strategies, and practical applications, check out our Further Reading section.
Have fun and happy path finding!
Further Reading
Core Concepts
-
Data Structures and Algorithms (DSA) - Complete Guide
A comprehensive collection of solutions and implementations for all fundamental algorithms and data structures. Includes detailed tutorials on sorting algorithms (bubble sort, merge sort, quick sort), searching algorithms (binary search, linear search), tree traversals, graph algorithms, and more. Serves as a central hub linking to in-depth explanations and implementations for each topic.
-
Floyd–Warshall Algorithm: A Comprehensive Guide to All-Pairs Shortest Paths
An in-depth guide to the Floyd–Warshall algorithm, covering its theoretical foundations, dynamic programming approach, and ability to compute all-pairs shortest paths efficiently. This guide includes pseudocode, mathematical background, performance analysis, and step-by-step implementations in Python, Java, C++, and JavaScript. Additionally, it explores detecting negative cycles and practical applications in network routing, computational geometry, and graph analysis.
-
A Note on Two Problems in Connexion with Graphs
Original 1959 paper by Edsger W. Dijkstra introducing the algorithm and its fundamental concepts.
Implementation Resources
-
NetworkX Library
Popular Python library implementing various graph algorithms, including an optimized version of Dijkstra's algorithm for real-world applications.
-
JGraphT
Java library offering high-performance implementations of graph algorithms and data structures, with extensive documentation and examples.
-
Boost Graph Library (BGL)
Comprehensive C++ library providing efficient implementations of graph algorithms and various optimization techniques.
Advanced Applications
-
Hierarchical Hub Labeleling for Shortest Paths
Research on optimizing shortest path algorithms for large-scale networks using advanced preprocessing techniques.
-
Engineering Multi-Level Overlay Graphs for Shortest-Path Queries
Advanced techniques for handling large-scale graph data with improved query performance.
Performance Optimization
-
Graph Algorithms: Advanced Optimization Techniques
In-depth coverage of performance optimization strategies for graph algorithms, including Dijkstra's algorithm variants.
-
Parallel Breadth-First Search and Exact Shortest Paths and Stronger Notions for Approximate Distances
This paper introduces new techniques for computing stronger approximations of shortest path distances, leading to the first efficient parallel algorithm for exact single-source shortest paths in directed graphs with n1/2+o(1) depth, resolving a long-standing open problem in parallel computing.
Attribution and Citation
If you found this guide and tools helpful, feel free to link back to this page or cite it in your work!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.