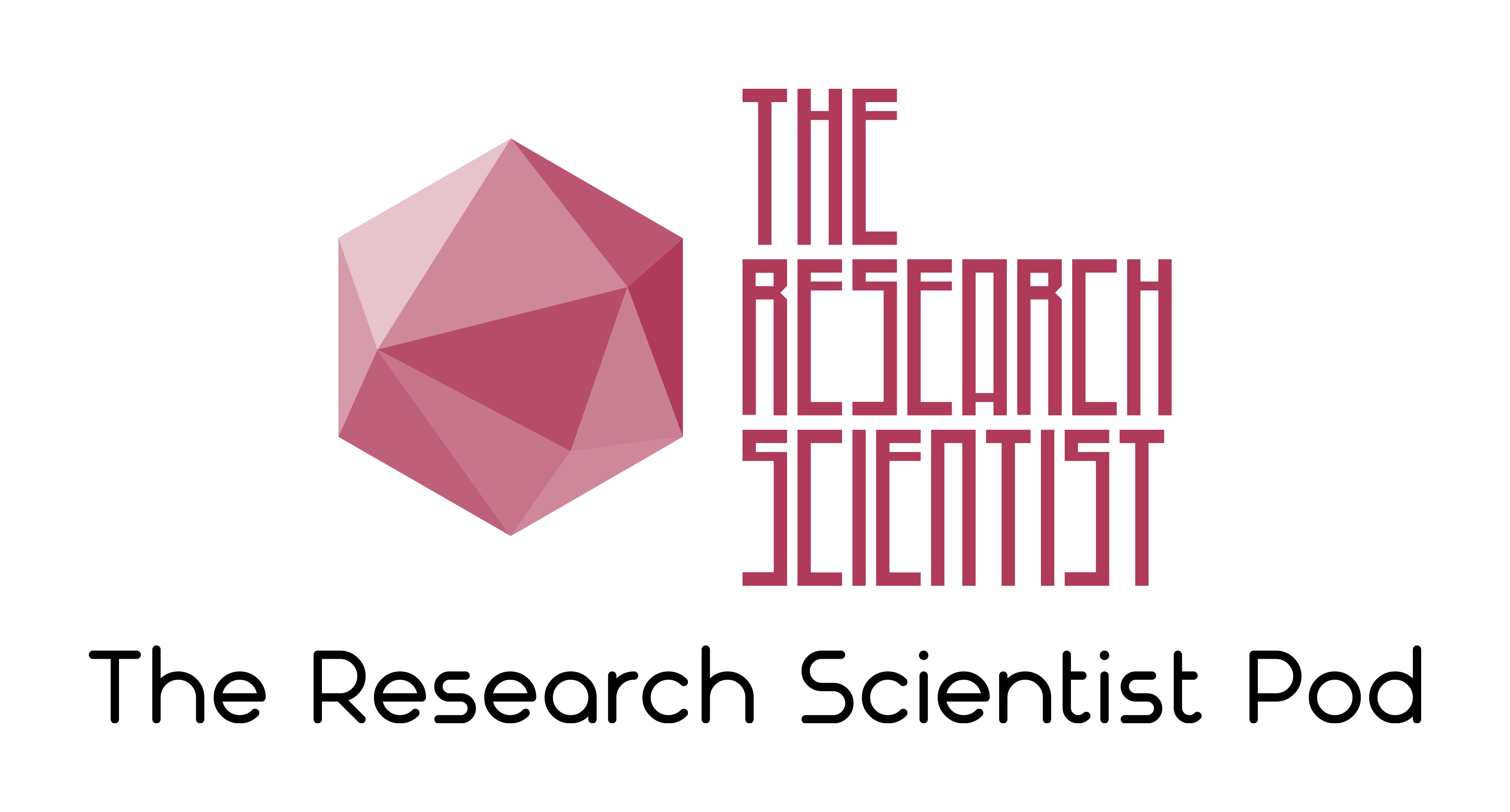
Levene's Test Results:
Understanding Levene's Test for Equality of Variances
Levene's Test is a statistical test used to assess whether two or more groups have equal variances. It is commonly applied in hypothesis testing scenarios to verify the assumption of homogeneity of variances, which is often a prerequisite for various parametric tests.
Key Components of Levene's Test
- Group Means or Medians: The central tendency (mean or median) of each group is used as a reference point for calculating deviations.
- Absolute Deviations: The deviations of each data point from its group mean or median are taken as absolute values, which form the basis of the test.
- Degrees of Freedom: Levene's Test uses degrees of freedom calculated as the number of groups minus one (for between-group variance) and total observations minus the number of groups (for within-group variance).
Formula for Levene's Test
The formula for Levene's Test depends on the absolute deviations from the group means (or medians) and calculates the F-statistic:
where:
- \( Z_{ij} \): Absolute deviation of the \(i\)-th observation in group \(j\) from the group mean or median.
- \( \bar{Z}_j \): Mean of absolute deviations for group \(j\).
- \( \bar{Z} \): Mean of all absolute deviations across groups.
- \( n_j \): Sample size of group \(j\).
- \( k \): Number of groups.
- \( N \): Total number of observations across all groups.
Programmatically Calculating Levene's Test
Below are examples for calculating Levene's Test in JavaScript, Python, and R.
1. Using JavaScript (with jStat)
In JavaScript, you can use the jStat library to calculate the components of Levene's Test:
Using Means
const group1 = [10, 15, 14, 18, 20];
const group2 = [12, 17, 16, 19, 21];
// Calculate means for each group (for standard Levene's test)
const mean1 = group1.reduce((a, b) => a + b, 0) / group1.length;
const mean2 = group2.reduce((a, b) => a + b, 0) / group2.length;
// Calculate absolute deviations from means
const deviations1 = group1.map(x => Math.abs(x - mean1));
const deviations2 = group2.map(x => Math.abs(x - mean2));
// Calculate mean deviations for each group
const meanDev1 = deviations1.reduce((a, b) => a + b, 0) / deviations1.length;
const meanDev2 = deviations2.reduce((a, b) => a + b, 0) / deviations2.length;
// Calculate overall mean deviation
const allDeviations = [...deviations1, ...deviations2];
const overallMeanDev = allDeviations.reduce((a, b) => a + b, 0) / allDeviations.length;
// Degrees of freedom
const dfBetween = 2 - 1; // number of groups minus 1
const dfWithin = group1.length + group2.length - 2; // total observations minus number of groups
// Calculate Sum of Squares Between Groups (SSB)
const SSB = group1.length * Math.pow(meanDev1 - overallMeanDev, 2) +
group2.length * Math.pow(meanDev2 - overallMeanDev, 2);
// Calculate Sum of Squares Within Groups (SSW)
const SSW = deviations1.reduce((sum, dev) => sum + Math.pow(dev - meanDev1, 2), 0) +
deviations2.reduce((sum, dev) => sum + Math.pow(dev - meanDev2, 2), 0);
// Calculate Mean Squares
const MSB = SSB / dfBetween;
const MSW = SSW / dfWithin;
// Calculate F-statistic
const fStatistic = MSB / MSW;
// Calculate p-value
const pValue = jStat.ftest(fStatistic, dfBetween, dfWithin);
console.log(`F-Statistic: ${fStatistic.toFixed(4)}`);
console.log(`p-value: ${pValue.toFixed(4)}`);
Using Medians
const group1 = [10, 15, 14, 18, 20];
const group2 = [12, 17, 16, 19, 21];
// Calculate medians for each group
const median1 = group1.sort((a,b) => a-b)[Math.floor(group1.length/2)];
const median2 = group2.sort((a,b) => a-b)[Math.floor(group2.length/2)];
// Calculate absolute deviations from medians
const deviations1 = group1.map(x => Math.abs(x - median1));
const deviations2 = group2.map(x => Math.abs(x - median2));
// Calculate mean deviations for each group
const meanDev1 = deviations1.reduce((a, b) => a + b, 0) / deviations1.length;
const meanDev2 = deviations2.reduce((a, b) => a + b, 0) / deviations2.length;
// Calculate overall mean deviation
const allDeviations = [...deviations1, ...deviations2];
const overallMeanDev = allDeviations.reduce((a, b) => a + b, 0) / allDeviations.length;
// Degrees of freedom
const dfBetween = 2 - 1; // number of groups minus 1
const dfWithin = group1.length + group2.length - 2; // total observations minus number of groups
// Calculate Sum of Squares Between Groups (SSB)
const SSB = group1.length * Math.pow(meanDev1 - overallMeanDev, 2) +
group2.length * Math.pow(meanDev2 - overallMeanDev, 2);
// Calculate Sum of Squares Within Groups (SSW)
const SSW = deviations1.reduce((sum, dev) => sum + Math.pow(dev - meanDev1, 2), 0) +
deviations2.reduce((sum, dev) => sum + Math.pow(dev - meanDev2, 2), 0);
// Calculate Mean Squares
const MSB = SSB / dfBetween;
const MSW = SSW / dfWithin;
// Calculate F-statistic
const fStatistic = MSB / MSW;
// Calculate p-value
const pValue = jStat.ftest(fStatistic, dfBetween, dfWithin);
console.log(`F-Statistic: ${fStatistic.toFixed(4)}`);
console.log(`p-value: ${pValue.toFixed(4)}`);
2. Using Python
In Python, the scipy.stats.levene
function directly performs Levene's Test:
from scipy.stats import levene
# Define data groups
group1 = [10, 15, 14, 18, 20]
group2 = [12, 17, 16, 19, 21]
# Perform Levene's Test
stat, p_value = levene(group1, group2, center='mean')
print(f"F-Statistic: {stat:.4f}, p-value: {p_value:.4f}")
You can change switch to using medians for the Levene's test by changing to center='median'
3. Using R
In R, you can use the leveneTest
function from the car
package:
library(car)
# Define data groups
group1 <- c(10, 15, 14, 18, 20)
group2 <- c(12, 17, 16, 19, 21)
# Perform Levene's Test
leveneTest(c(group1, group2) ~ factor(rep(1:2, each = length(group1))), center = median)
You can change switch to using medians for the Levene's test by changing to center='median'