Introduction
In this post, we’ll explore Binary Search Insertion, a variation of binary search that allows you to insert elements into a sorted array efficiently. While binary search is typically used for searching through sorted data, binary search insertion extends this functionality by maintaining the sorted order even as new elements are dynamically added.
We’ll explain the advantages of binary search insertion and the code implementations in Python, C++, Java, and JavaScript. We will also discuss its time and space complexity, use cases, optimizations, and scenarios where it is not applicable.
How Binary Search Insertion Works
Binary search insertion combines two processes: first, a binary search is used to identify the correct position for the new element, and then an insertion step shifts elements as needed to accommodate the new element while maintaining the sorted order.
Step-by-Step Process
- Binary Search Phase:
- Use binary search to find the correct insertion point for the new element. Start by comparing the element with the middle element of the array.
- If the new element is smaller than the middle element, continue searching the left half; if it is larger, search the right half.
- The process repeats until the correct insertion index is identified.
- Insertion Phase:
- Once the insertion point is found, shift all elements after this index to the right by one position to make room for the new element.
- Insert the new element at the identified index.
Time Complexity
The binary search takes O(log n) time, but the insertion phase, which involves shifting elements, can take O(n) time in the worst case. Therefore, the insertion step dominates the overall time complexity, resulting in an O(n) worst-case complexity.
Practical Considerations
In practice, binary search insertion is often used in scenarios where the array is already mostly sorted and only occasional insertions are needed. In such cases, the average-case performance can be better than O(n), especially if insertions occur near the array’s end.
While the worst-case time complexity remains O(n) due to the potential need to shift many elements, the algorithm can be more efficient in real-world applications where the data structure is frequently queried and occasionally updated.
Example:
Let’s walk through a quick example with the sorted array: arr = [1, 3, 5, 7, 9]
You want to insert the value 6
:
- Binary Search: The middle element is
5
. Since6 > 5
, search in the right half ([7, 9]
). - Binary Search: Compare
6
with7
. Since6 < 7
, the insertion index is between5
and7
. - Insert Element: Shift
7
and9
to the right, and insert6
at index 3. The new array becomes[1, 3, 5, 6, 7, 9]
.
Below is a visualization tool to see how binary search insertion performs in real-time.
This approach ensures that the array remains sorted after insertion while using Binary Search to minimize the time it takes to find the appropriate position.
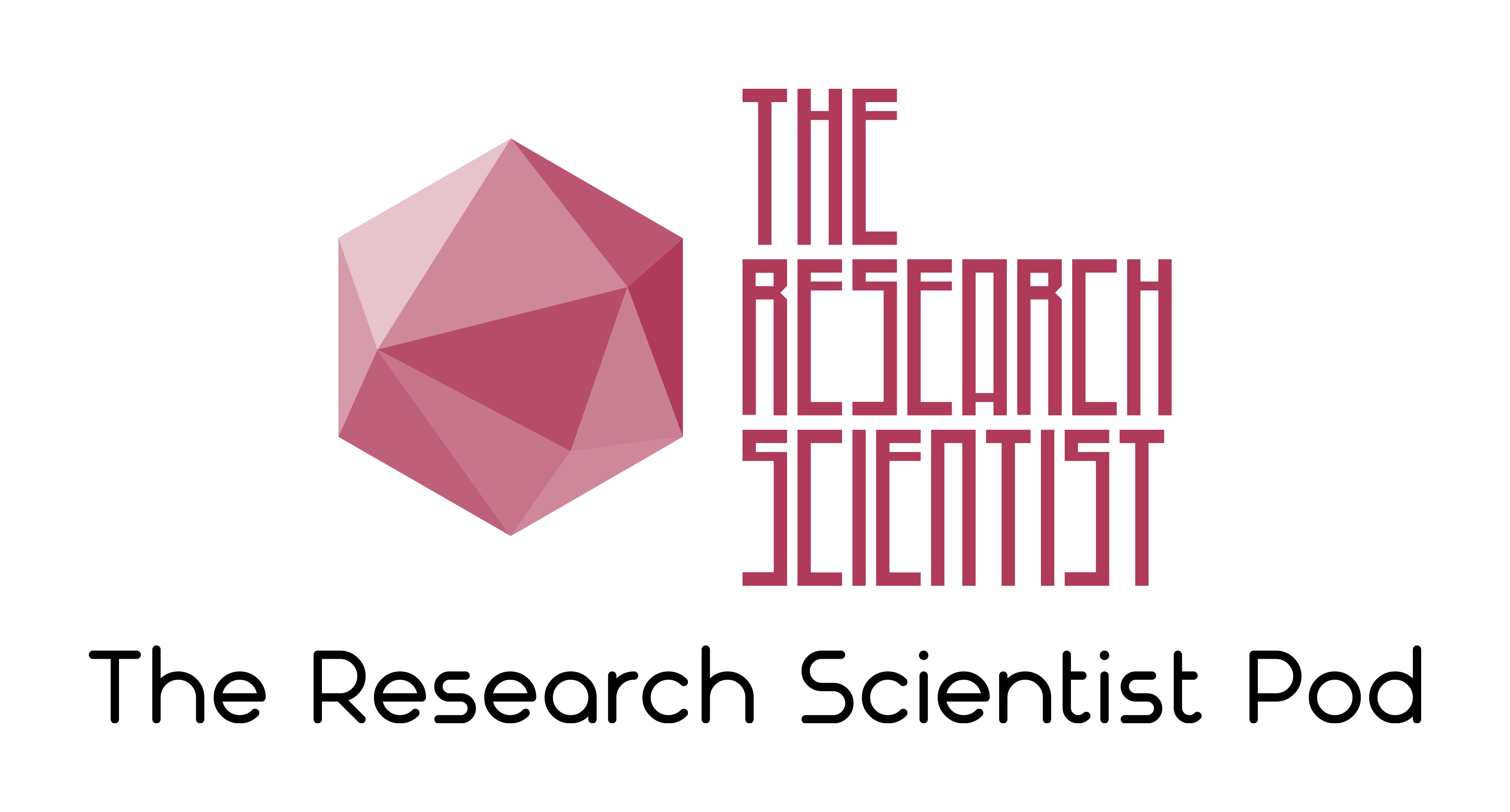
Recursive vs Iterative Implementations of Binary Search Insertion
Binary search insertion can be tackled using either a recursive or iterative approach, each with its unique advantages and trade-offs.
Recursive Implementation
- Follows a divide-and-conquer strategy intuitively, splitting the array and calling itself until the correct insertion point is found.
- Adds overhead due to function calls and uses O(log n) space because of additional stack frames.
- It is conceptually more straightforward for some developers to understand.
Iterative Implementation
- It avoids recursion overhead by using a loop to find the correct insertion point.
- More memory efficient, using O(1) space for the binary search part.
- Often preferred for large datasets where recursion might cause a stack overflow.
Comparison
- Both approaches have the same time complexity of O(log n) for binary search and O(n) overall due to the insertion step.
- The iterative method is more efficient in real-world scenarios due to its lower space complexity and absence of function call overhead.
- The choice between recursive and iterative depends on project requirements: readability, memory constraints, and performance needs.
Important Notes
- Both require O(n) space in total due to the array insertion nature.
- The binary search part (recursive or iterative) has a time complexity of O(log n).
- The overall time complexity of O(n) comes from the insertion step.
- The recursive approach uses O(log n) space for binary search, while the iterative uses O(1) space.
Code Implementation in Different Languages
Let’s examine how Binary Search can be combined with the insertion process in various programming languages.
def binary_search_insert(arr, target):
left, right = 0, len(arr) - 1
# Use binary search to find the correct insertion point
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid # Insert at the mid position
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
# Insert the target at the correct position
arr.insert(left, target) # 'left' is the correct insertion point
arr = [1, 3, 5, 7, 9]
binary_search_insert(arr, 6)
print(arr) # Output: [1, 3, 5, 6, 7, 9]
#include <iostream>
#include <vector>
void binarySearchInsert(std::vector<int>& arr, int target) {
int left = 0, right = arr.size() - 1;
// Binary search for the correct position
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
left = mid; // Insert at mid
break;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
// Insert the target at the correct position
arr.insert(arr.begin() + left, target);
}
int main() {
std::vector<int> arr = {1, 3, 5, 7, 9};
binarySearchInsert(arr, 6);
for (int x : arr)
std::cout << x << " ";
// Output: 1 3 5 6 7 9
}
import java.util.ArrayList;
import java.util.List;
public class BinarySearchInsert {
public static void binarySearchInsert(List<Integer> arr, int target) {
int left = 0, right = arr.size() - 1;
// Binary search for the correct insertion point
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr.get(mid) == target) {
left = mid;
break;
} else if (arr.get(mid) < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
// Insert the target at the correct position
arr.add(left, target);
}
public static void main(String[] args) {
List<Integer> arr = new ArrayList<>(List.of(1, 3, 5, 7, 9));
binarySearchInsert(arr, 6);
System.out.println(arr); // Output: [1, 3, 5, 6, 7, 9]
}
}
function binarySearchInsert(arr, target) {
let left = 0, right = arr.length - 1;
// Use binary search to find the insertion point
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
left = mid; // Insert at mid position
break;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
// Insert the target at the correct position
arr.splice(left, 0, target);
}
let arr = [1, 3, 5, 7, 9];
binarySearchInsert(arr, 6);
console.log(arr); // Output: [1, 3, 5, 6, 7, 9]
Use Cases of Binary Search Insertion
Binary search insertion is ideal in scenarios where a sorted list or array must be maintained dynamically as new data arrives. Some key use cases include:
Maintaining a Sorted List or Array:
- Binary search insertion is highly effective whenever a dataset needs to remain sorted after frequent insertions. This could apply to maintaining a priority queue, leaderboard, or any collection where order is important.
- Example: Inserting player scores in a sorted order in a game leaderboard.
Real-Time Financial Systems:
- Maintaining a sorted list of transactions or orders in stock market trading or financial applications is vital for quick lookups. Binary search insertion inserts new data (such as stock prices or transaction amounts) into sorted arrays while keeping the dataset ordered.
- Example: Maintaining a sorted list of stock prices for quick retrieval of highest/lowest values.
Data Deduplication:
- When inserting elements into a sorted array where duplicates are not allowed, binary search can first verify if the element already exists. If not, it inserts the element into the correct position.
- Example: Maintaining a list of unique user IDs or unique product names.
Scheduling Systems:
- Real-time scheduling algorithms often require tasks to be inserted into a list based on deadlines or priorities. Binary search insertion ensures that the task list remains sorted, allowing for efficient scheduling decisions.
- Example: Inserting new tasks into a priority-based task scheduler.
Database Indexing:
- While not typically used in large-scale database systems, the principles of binary search insertion can be applied in simpler database implementations or in-memory indices to maintain sorted keys for faster lookups.
Autocomplete and Search Suggestions:
- In systems that provide autocomplete functionality, binary search insertion can be used to maintain a sorted list of frequently used or trending search terms.
- Example: Updating a list of popular search terms as users enter new queries.
Version Control Systems:
- When managing different versions of files or documents, binary search insertion can be used to maintain a sorted list of version numbers or timestamps.
Important Considerations:
- While binary search insertion is efficient for small to medium-sized datasets, more advanced data structures like self-balancing trees (e.g., AVL trees, Red-Black trees) or B-trees might be more appropriate for very large datasets or high-frequency insertions.
- The efficiency of binary search insertion can degrade if many insertions occur at the beginning of the array, as this requires shifting many elements. In such cases, alternative data structures might be considered.
Edge Cases and Pitfalls in Binary Search Insertion
When implementing binary search insertion, several edge cases and potential pitfalls should be considered:
Empty Array:
- The simplest edge case occurs when the array is empty. In this case, the element is inserted as the first element.
- The binary search logic should handle this gracefully by recognizing an empty array and skipping the search phase.
Duplicate Elements:
- If duplicates are allowed in the array, binary search insertion can place the new element at the first occurrence of its duplicate.
- In some applications, you may want to insert it at the last occurrence or avoid inserting duplicates altogether.
- The implementation should clearly define and consistently handle the behaviour of duplicates.
Array Capacity Limits:
- In languages like C++ or Java, where arrays have a fixed size, exceeding the array’s capacity can cause errors or undefined behaviour.
- It’s important to handle dynamic resizing or to ensure the array has enough capacity before insertion.
- Consider using dynamic data structures like ArrayList in Java or vector in C++ for automatic resizing.
Cost of Shifting Elements:
- For very large arrays, the cost of shifting elements during insertion can become expensive.
- While binary search reduces the time required to find the insertion point, shifting elements still takes O(n) time.
- Other data structures, like balanced binary search trees, may perform better if frequent insertions are required.
Integer Overflow:
- When calculating the middle index in binary search (e.g.,
mid = (left + right) / 2
), integer overflow can occur for very large arrays. - A safer approach is to use
mid = left + (right - left) / 2
to avoid this issue.
Handling of Boundaries:
- Particular attention should be paid to handling array boundaries during the binary search phase.
- Ensure that the algorithm correctly handles cases where the element should be inserted at the beginning or end of the array.
Performance Degradation with Sorted Input:
- Suppose the input is already sorted or nearly sorted, and elements are frequently inserted at the beginning of the array. In that case, the performance can degrade to O(n) for each insertion due to the need to shift elements.
- In such cases, consider alternative data structures or insertion strategies.
Concurrency Issues:
- In multi-threaded environments, concurrent insertions can lead to race conditions and data inconsistencies.
- Proper synchronization mechanisms should be implemented if multiple threads access the data structure.
Important Note:
While binary search insertion is efficient for many use cases, it’s crucial to consider your application’s specific requirements. For scenarios involving frequent insertions or extremely large datasets, more advanced data structures like self-balancing trees (e.g., AVL trees, Red-Black trees), or B-trees might be more appropriate.
When Binary Search Insertion is Not Applicable
While binary search insertion is effective for maintaining sorted arrays, it’s not always the best choice:
Unsorted Data:
- Binary search requires sorted data. For unsorted data, binary search insertion fails.
- Sorting first takes O(n log n) time, negating binary search benefits.
- Alternative: Use a heap or priority queue (O(log n) insertion) for unsorted data with frequent insertions.
Highly Dynamic Data:
- Balanced binary search trees excel for frequent insertions and removals with critical order maintenance.
- Trees like AVL or Red-Black offer O(log n) insertion without element shifting.
- Alternative: Use self-balancing trees for datasets requiring frequent modifications.
Linked Lists:
- Binary search insertion is impractical for linked lists due to a lack of random access.
- Accessing the middle of a linked list takes O(n) time, negating binary search advantages.
- Alternative: Use linear search for insertion in linked lists.
Large-Scale Data:
- For vast datasets, O(n) element shifting cost may be prohibitive.
- Alternatives: Consider balanced search trees, skip lists, or hash tables for better scalability.
Continuous Real-Time Insertions:
- In systems requiring constant, high-frequency insertions, the shifting cost may cause performance issues.
- Alternative: Use data structures allowing O(1) or amortized O(1) insertions, like hash tables or dynamic arrays with occasional rebalancing.
Memory-Constrained Environments:
- Binary search insertion in arrays requires contiguous memory allocation.
- In memory-limited systems, this can be problematic for large or growing datasets.
- Alternative: Use linked structures or memory-efficient trees.
Multi-Dimensional Data:
- Binary search insertion is designed for one-dimensional data.
- For multi-dimensional datasets requiring sorted storage, it’s not directly applicable.
- Alternative: Consider space-partitioning data structures like k-d trees or R-trees.
Important note:
When choosing a data structure, always consider your application’s specific requirements, including insertion/deletion frequency, query patterns, and scalability needs.
Optimizations for Binary Search Insertion
While binary search insertion is efficient, several optimizations can further improve its performance:
Batch Insertion:
- Sort and merge new elements with the existing sorted array instead of inserting them individually.
- Reduces element-shifting overhead; each batch can merge in O(n) time.
- Example: Inserting multiple transactions into a sorted financial record in one pass.
Self-Balancing Trees:
- Use balanced binary search trees (e.g., AVL or Red-Black trees) for frequent insertions in large datasets.
- Offers O(log n) insertion time without element shifting.
- Maintains balance automatically, ensuring consistent performance.
Dynamic Arrays Management:
- Preallocate space or use amortised resizing in languages with dynamic arrays (e.g., Python).
- Reduces performance degradation from frequent resizing.
- When resizing is necessary, consider implementing a growth factor (e.g., 1.5x or 2x).
Binary Insertion Sort:
- For small arrays or partially sorted data, combine binary search with insertion sort.
- Use binary search to find the insertion point, then use insertion sort’s shifting mechanism.
- It can be more cache-friendly for small datasets.
Cache-Aware Implementations:
- Design the algorithm to be cache-friendly, minimizing cache misses.
- Consider the size of cache lines when deciding how to structure data and perform operations.
- Use techniques like loop unrolling or blocking to improve cache utilization.
Adaptive Binary Search:
- Implement an adaptive strategy that switches between binary and linear search based on the size of the search range.
- For small ranges, linear search can outperform binary search due to its simplicity and cache-friendly nature.
Parallel Processing:
- For very large datasets, implement parallel processing techniques.
- Divide the array into sections and perform a binary search on each section concurrently.
- Merge the results to determine the final insertion point.
Hybrid Data Structures:
- Combine binary search insertion with other data structures for specific use cases.
- Example: Use a combination of arrays and linked lists (skip lists) for efficient insertion and search.
Memory Pool Allocation:
- Use a memory pool to manage system allocations with frequent insertions and deletions.
- Reduces overhead from frequent memory allocation and deallocation.
Custom Comparison Functions:
- Implement efficient comparison functions tailored to your specific data types.
- It can significantly improve performance, especially for complex objects.
Important Note:
The effectiveness of these optimizations depends on your specific use case, data characteristics, and system constraints. Profile your application to identify bottlenecks and apply optimizations judiciously.
Conclusion
Binary search insertion is an efficient technique for maintaining sorted arrays and lists while dynamically inserting new elements. Leveraging binary search to find the correct insertion point significantly reduces the time complexity compared to linear insertion. Although its worst-case time complexity is O(n) due to the element shifting step, it remains highly effective for scenarios that require maintaining sorted order, such as real-time scheduling, financial systems, or game development.
While binary search insertion excels in many cases, it’s important to recognize its limitations. Other data structures like balanced search trees or priority queues might be more appropriate for unsorted data, frequent insertions, or large datasets. Mastering binary search insertion and understanding its trade-offs will help you choose the right tool for your data management needs.
Congratulations on reading to the end of this tutorial!
For further reading on binary search, go to the article: Binary Search: A Comprehensive Guide
For further reading on data structures and algorithms, please visit the DSA page!
Have fun and happy researching!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.