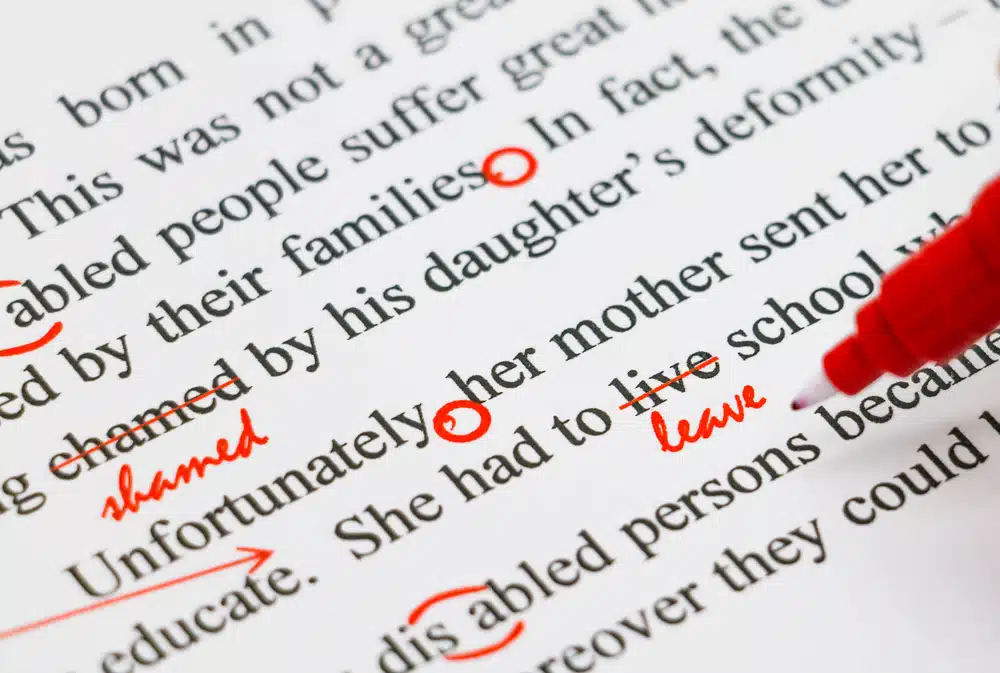
Welcome to our comprehensive guide on the Levenshtein distance algorithm, a fundamental metric in string comparison and text processing. In this article, we’ll explore how this powerful algorithm works, its implementation across different programming languages, and its practical applications.
Table of Contents
Introduction
The Levenshtein distance, also known as edit distance, is a string metric that measures the minimum number of single-character operations required to transform one string into another. Named after Vladimir Levenshtein, who introduced it in 1965, this algorithm has become a cornerstone in various applications, from spell-checking to DNA sequence analysis.
Key Features
- Measures the minimum number of edits needed to transform one string into another
- Considers insertions, deletions, and substitutions
- Provides a numeric measure of string dissimilarity
- Symmetric property: distance(s1, s2) = distance(s2, s1)
Mathematical Background
The Levenshtein distance uses dynamic programming to compute the minimum number of single-character edits required to transform one string into another. Let’s break down the mathematical foundations and understand how the algorithm works.
Core Concept
For two strings \(a\) and \(b\) of length \(|a|\) and \(|b|\), the Levenshtein distance \(lev_{a,b}(i,j)\) between the first \(i\) characters of \(a\) and the first \(j\) characters of \(b\) is defined as:
\[ lev_{a,b}(i,j) = \begin{cases} \max(i,j) & \text{if } \min(i,j) = 0 \\ \min \begin{cases} lev_{a,b}(i-1,j) + 1 \\ lev_{a,b}(i,j-1) + 1 \\ lev_{a,b}(i-1,j-1) + 1_{(a_i \neq b_j)} \end{cases} & \text{otherwise} \end{cases} \]where:
- \(1_{(a_i \neq b_j)}\) is the indicator function, equal to 0 when \(a_i = b_j\) and 1 otherwise
- The first case represents the distance if one string is empty
- The three options in the second case represent deletion, insertion, and substitution respectively
Understanding the Operations
- Insertion (Cost = 1): Add a character to match the target string
- Deletion (Cost = 1): Remove a character to match the target string
- Substitution (Cost = 1): Replace one character with another
Dynamic Programming Matrix
The algorithm constructs a matrix \(D\) where \(D[i,j]\) represents the Levenshtein distance between the first \(i\) characters of string \(a\) and the first \(j\) characters of string \(b\).
Let’s calculate the Levenshtein distance between “KITTEN” and “SITTING”. This measures the minimum number of single-character edits needed to transform one string into another.
Ø | S | I | T | T | I | N | G | |
---|---|---|---|---|---|---|---|---|
Ø | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
K | 1 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
I | 2 | 2 | 1 | 2 | 3 | 4 | 5 | 6 |
T | 3 | 3 | 2 | 1 | 2 | 3 | 4 | 5 |
T | 4 | 4 | 3 | 2 | 1 | 2 | 3 | 4 |
E | 5 | 5 | 4 | 3 | 2 | 2 | 3 | 4 |
N | 6 | 6 | 5 | 4 | 3 | 3 | 2 | 3 |
Final Distance = 3
The optimal path (highlighted in green) shows the minimum operations needed:
- Replace ‘K’ with ‘S’ (substitution)
- Replace ‘E’ with ‘I’ (substitution)
- Insert ‘G’ at the end (insertion)
Hover over any cell to see the operation it represents!
Properties and Considerations
-
Triangle Inequality:
Formula: \( lev(a, c) \leq lev(a, b) + lev(b, c) \)
This means that the distance between two words \(a\) and \(c\) is always less than or equal to the sum of the distances from \(a\) to \(b\) and from \(b\) to \(c\). In simple terms, if you are transforming \(a\) into \(c\), going through an intermediate word \(b\) will never take fewer edits than the direct transformation. This ensures consistency and logical transformations.
-
Symmetry:
Formula: \( lev(a, b) = lev(b, a) \)
The distance between two words \(a\) and \(b\) is the same regardless of the order. For example, transforming “cat” into “bat” takes the same number of edits as transforming “bat” into “cat.” This reflects the fairness of the metric, as it doesn’t favor one direction over the other.
-
Non-negativity:
Formula: \( lev(a, b) \geq 0 \)
The Levenshtein distance is always zero or greater. Negative distances are impossible because the distance represents the count of edits (insertions, deletions, or substitutions), which cannot be negative.
-
Bounded:
Formula: \( lev(a, b) \leq \max(|a|, |b|) \)
The Levenshtein distance between two words \(a\) and \(b\) can never exceed the length of the longer word. At most, you would need to delete all characters from one word and insert all characters from the other. For example, transforming “cat” into “elephant” would require removing all three letters in “cat” and adding all eight letters of “elephant,” resulting in a distance of 8.
-
Identity:
Formula: \( lev(a, b) = 0 \text{ if and only if } a = b \)
The distance between two words \(a\) and \(b\) is zero only if the two words are identical. If there is any difference between the two, the distance will always be greater than zero. For example, the distance between “apple” and “apple” is 0 because no edits are needed.
Time and Space Complexity
The standard dynamic programming implementation has:
- Time Complexity: \(O(mn)\) where \(m\) and \(n\) are the lengths of the input strings
- Space Complexity: \(O(mn)\) for the full matrix, or \(O(\min(m,n))\) if only the previous row is stored
Variations and Extensions
- Damerau-Levenshtein distance: Also considers transposition of adjacent characters
- Weighted Levenshtein distance: Assigns different costs to different operations
- Restricted edit distance: Limits the types of operations allowed
Implementation
The Levenshtein distance algorithm is a powerful tool for measuring the similarity between strings. We’ll explore implementations in Python, R, and C++, each offering unique advantages while maintaining the core dynamic programming approach. Each implementation includes both a full matrix version for educational purposes and a space-efficient version for practical applications.
Implementation Strategy
Our implementations follow these key principles:
- Dynamic programming matrix for optimal subproblem solving and efficient computation
- Space optimization through row-by-row computation
- Comprehensive error handling and edge case management
- Clear documentation with type hints where language-appropriate
Complete Implementation
Python Implementation
The Python implementation leverages NumPy for efficient matrix operations while maintaining readability. It provides both a full matrix implementation for visualization and a space-efficient version for production use.
- Type hints ensure code maintainability
- NumPy integration for optimized matrix operations
- Comprehensive docstrings for clear documentation
from typing import List, Tuple
import numpy as np
def levenshtein_distance(s1: str, s2: str) -> Tuple[int, List[List[int]]]:
"""
Calculate the Levenshtein distance between two strings and return the distance
matrix for backtracking.
Args:
s1 (str): First string
s2 (str): Second string
Returns:
Tuple[int, List[List[int]]]: (distance, matrix)
"""
rows, cols = len(s1) + 1, len(s2) + 1
matrix = np.zeros((rows, cols), dtype=int)
# Initialize first row and column
matrix[0, :] = np.arange(cols)
matrix[:, 0] = np.arange(rows)
# Fill matrix using vectorized operations where possible
for i in range(1, rows):
for j in range(1, cols):
if s1[i-1] == s2[j-1]:
matrix[i, j] = matrix[i-1, j-1]
else:
matrix[i, j] = min(
matrix[i-1, j] + 1, # deletion
matrix[i, j-1] + 1, # insertion
matrix[i-1, j-1] + 1 # substitution
)
return matrix[rows-1, cols-1], matrix.tolist()
def space_efficient_levenshtein(s1: str, s2: str) -> int:
"""
Calculate Levenshtein distance using O(min(len(s1), len(s2))) space.
"""
if len(s1) > len(s2):
s1, s2 = s2, s1
previous_row = list(range(len(s2) + 1))
current_row = [0] * (len(s2) + 1)
for i in range(1, len(s1) + 1):
current_row[0] = i
for j in range(1, len(s2) + 1):
if s1[i-1] == s2[j-1]:
current_row[j] = previous_row[j-1]
else:
current_row[j] = min(
previous_row[j] + 1, # deletion
current_row[j-1] + 1, # insertion
previous_row[j-1] + 1 # substitution
)
previous_row, current_row = current_row, previous_row
return previous_row[-1]
# Example usage and visualization
def visualize_alignment(s1: str, s2: str, matrix: List[List[int]]) -> str:
"""
Visualize the optimal alignment between two strings based on the Levenshtein matrix.
"""
i, j = len(s1), len(s2)
alignment = []
while i > 0 or j > 0:
if i > 0 and j > 0 and s1[i-1] == s2[j-1]:
alignment.append((s1[i-1], s2[j-1], "match"))
i -= 1
j -= 1
elif i > 0 and (j == 0 or matrix[i][j] == matrix[i-1][j] + 1):
alignment.append((s1[i-1], "-", "deletion"))
i -= 1
elif j > 0 and (i == 0 or matrix[i][j] == matrix[i][j-1] + 1):
alignment.append(("-", s2[j-1], "insertion"))
j -= 1
else:
alignment.append((s1[i-1], s2[j-1], "substitution"))
i -= 1
j -= 1
alignment.reverse()
return alignment
# Example with full matrix
s1, s2 = "kitten", "sitting"
distance, matrix = levenshtein_distance(s1, s2)
print(f"Distance between '{s1}' and '{s2}': {distance}")
# Space-efficient version
efficient_distance = space_efficient_levenshtein(s1, s2)
print(f"Space-efficient distance: {efficient_distance}")
alignment = visualize_alignment(s1, s2, matrix)
for a, b, op in alignment:
print(f"{a:2} → {b:2} ({op})")
Example Usage:
Distance between 'kitten' and 'sitting': 3 Space-efficient distance: 3 k → s (substitution) i → i (match) t → t (match) t → t (match) e → i (substitution) n → n (match) - → g (insertion)
R Implementation
The R implementation emphasizes clarity and vectorized operations while maintaining excellent performance. It includes helper functions for visualization and analysis.
- Efficient matrix operations
- Clear variable naming and structure
- Comprehensive error handling
levenshtein_distance <- function(s1, s2) {
# Input validation
if (!is.character(s1) || !is.character(s2)) {
stop("Inputs must be character strings")
}
# Create matrix
rows <- nchar(s1) + 1
cols <- nchar(s2) + 1
matrix <- matrix(0, nrow = rows, ncol = cols)
# Initialize efficiently
matrix[1, ] <- 0:(cols-1)
matrix[, 1] <- 0:(rows-1)
# Split strings once for efficiency
s1_chars <- strsplit(s1, "")[[1]]
s2_chars <- strsplit(s2, "")[[1]]
# Main computation
for (i in 2:rows) {
for (j in 2:cols) {
if (s1_chars[i-1] == s2_chars[j-1]) {
matrix[i, j] <- matrix[i-1, j-1]
} else {
matrix[i, j] <- min(
matrix[i-1, j] + 1, # deletion
matrix[i, j-1] + 1, # insertion
matrix[i-1, j-1] + 1 # substitution
)
}
}
}
return(list(
distance = matrix[rows, cols],
matrix = matrix
))
}
space_efficient_levenshtein <- function(s1, s2) {
if (nchar(s1) > nchar(s2)) {
temp <- s1
s1 <- s2
s2 <- temp
}
s1_len <- nchar(s1)
s2_len <- nchar(s2)
previous_row <- 0:s2_len
current_row <- integer(s2_len + 1)
s1_chars <- strsplit(s1, "")[[1]]
s2_chars <- strsplit(s2, "")[[1]]
for (i in 1:s1_len) {
current_row[1] <- i
for (j in 1:s2_len) {
if (s1_chars[i] == s2_chars[j]) {
current_row[j + 1] <- previous_row[j]
} else {
current_row[j + 1] <- min(
previous_row[j + 1] + 1,
current_row[j] + 1,
previous_row[j] + 1
)
}
}
previous_row <- current_row
current_row <- integer(s2_len + 1)
}
return(previous_row[s2_len + 1])
}
visualize_alignment <- function(s1, s2, result) {
matrix <- result$matrix
i <- nrow(matrix)
j <- ncol(matrix)
alignment <- list()
while (i > 1 || j > 1) {
if (i > 1 && j > 1 && substr(s1, i-1, i-1) == substr(s2, j-1, j-1)) {
alignment[[length(alignment) + 1]] <- list(
c1 = substr(s1, i-1, i-1),
c2 = substr(s2, j-1, j-1),
op = "match"
)
i <- i - 1
j <- j - 1
} else if (i > 1 && (j == 1 || matrix[i,j] == matrix[i-1,j] + 1)) {
alignment[[length(alignment) + 1]] <- list(
c1 = substr(s1, i-1, i-1),
c2 = "-",
op = "deletion"
)
i <- i - 1
} else if (j > 1 && (i == 1 || matrix[i,j] == matrix[i,j-1] + 1)) {
alignment[[length(alignment) + 1]] <- list(
c1 = "-",
c2 = substr(s2, j-1, j-1),
op = "insertion"
)
j <- j - 1
} else {
alignment[[length(alignment) + 1]] <- list(
c1 = substr(s1, i-1, i-1),
c2 = substr(s2, j-1, j-1),
op = "substitution"
)
i <- i - 1
j <- j - 1
}
}
return(rev(alignment))
}
# Full matrix example
s1 <- "kitten"
s2 <- "sitting"
result <- levenshtein_distance(s1, s2)
print(sprintf("Distance between '%s' and '%s': %d",
s1, s2, result$distance))
# Space-efficient version
distance <- space_efficient_levenshtein(s1, s2)
print(sprintf("Space-efficient distance: %d", distance))
alignment <- visualize_alignment(s1, s2, result)
for (a in alignment) {
cat(sprintf("%s → %s (%s)\n", a$c1, a$c2, a$op))
}
Example Usage:
Distance between 'kitten' and 'sitting': 3 Space-efficient distance: 3 k → s (substitution) i → i (match) t → t (match) t → t (match) e → i (substitution) n → n (match) - → g (insertion)
C++ Implementation
The C++ implementation focuses on performance while maintaining clarity. It uses modern C++ features and STL containers for efficient memory management.
- Optimized memory allocation patterns
- Modern C++ features for clarity and safety
- Efficient use of STL containers
#include <vector>
#include <string>
#include <iostream>
#include <numeric>
#include <iomanip>
struct AlignmentStep {
char c1;
char c2;
std::string operation;
};
struct LevenshteinResult {
int distance;
std::vector<std::vector<int>> matrix;
};
std::vector<AlignmentStep> visualize_alignment(
const std::string& s1,
const std::string& s2,
const std::vector<std::vector<int>>& matrix) {
std::vector<AlignmentStep> alignment;
size_t i = s1.length();
size_t j = s2.length();
while (i > 0 || j > 0) {
if (i > 0 && j > 0 && s1[i-1] == s2[j-1]) {
alignment.push_back({
s1[i-1],
s2[j-1],
"match"
});
i--;
j--;
} else if (i > 0 && (j == 0 || matrix[i][j] == matrix[i-1][j] + 1)) {
alignment.push_back({
s1[i-1],
'-',
"deletion"
});
i--;
} else if (j > 0 && (i == 0 || matrix[i][j] == matrix[i][j-1] + 1)) {
alignment.push_back({
'-',
s2[j-1],
"insertion"
});
j--;
} else {
alignment.push_back({
s1[i-1],
s2[j-1],
"substitution"
});
i--;
j--;
}
}
std::reverse(alignment.begin(), alignment.end());
return alignment;
}
LevenshteinResult levenshtein_distance(const std::string& s1,
const std::string& s2) {
if (s1.empty() && s2.empty()) return {0, {{0}}};
const size_t rows = s1.length() + 1;
const size_t cols = s2.length() + 1;
std::vector matrix(rows, std::vector<int>(cols));
// Initialize efficiently
for (size_t i = 0; i < rows; ++i) matrix[i][0] = i;
for (size_t j = 0; j < cols; ++j) matrix[0][j] = j;
// Main computation
for (size_t i = 1; i < rows; ++i) {
for (size_t j = 1; j < cols; ++j) {
if (s1[i-1] == s2[j-1]) {
matrix[i][j] = matrix[i-1][j-1];
} else {
matrix[i][j] = std::min({
matrix[i-1][j] + 1, // deletion
matrix[i][j-1] + 1, // insertion
matrix[i-1][j-1] + 1 // substitution
});
}
}
}
return {matrix[rows-1][cols-1], std::move(matrix)};
}
int space_efficient_levenshtein(const std::string& s1,
const std::string& s2) {
const std::string* str1 = &s1;
const std::string* str2 = &s2;
if (s1.length() > s2.length()) {
std::swap(str1, str2);
}
const size_t str1_len = str1->length();
const size_t str2_len = str2->length();
std::vector<int> previous_row(str2_len + 1);
std::vector<int> current_row(str2_len + 1);
std::iota(previous_row.begin(), previous_row.end(), 0);
for (size_t i = 0; i < str1_len; ++i) {
current_row[0] = i + 1;
for (size_t j = 0; j < str2_len; ++j) {
if ((*str1)[i] == (*str2)[j]) {
current_row[j + 1] = previous_row[j];
} else {
current_row[j + 1] = std::min({
previous_row[j + 1] + 1, // deletion
current_row[j] + 1, // insertion
previous_row[j] + 1 // substitution
});
}
}
std::swap(previous_row, current_row);
}
return previous_row[str2_len];
}
int main() {
const std::string s1 = "kitten";
const std::string s2 = "sitting";
auto [distance, matrix] = levenshtein_distance(s1, s2);
std::cout << "Levenshtein distance between '" << s1
<< "' and '" << s2 << "': "
<< distance << "\n\n";
std::cout << "\nSpace-efficient calculation: "
<< space_efficient_levenshtein(s1, s2) << "\n";
std::cout << "Alignment:\n";
auto alignment = visualize_alignment(s1, s2, matrix);
for (const auto& step : alignment) {
std::cout << step.c1 << " → " << step.c2
<< " (" << step.operation << ")\n";
}
return 0;
}
Example Usage:
Levenshtein distance between 'kitten' and 'sitting': 3 Space-efficient calculation: 3 Alignment: k → s (substitution) i → i (match) t → t (match) t → t (match) e → i (substitution) n → n (match) - → g (insertion)
Performance Analysis
Understanding the performance characteristics of each implementation helps in choosing the right approach for your specific use case:
- Time Complexity: O(mn) for all implementations, where m and n are the string lengths
- Space Complexity:
- Full Matrix: O(mn)
- Space-Efficient: O(min(m,n))
- Memory Usage: The space-efficient versions use significantly less memory for long strings
- CPU Usage: The C++ implementation typically performs fastest, followed by Python with NumPy, then R
Implementation Tips
- Always normalize strings (case, whitespace) before comparison
- Use space-efficient version for production environments
- Consider using integer overflow protection for very long strings
- Implement proper error handling for edge cases
Examples and Applications
The Levenshtein distance algorithm finds practical applications across various domains, from spell-checking to bioinformatics. Let's explore some common use cases with practical implementations.
1. Spell Checking and Auto-Correction
Spell-checking and auto-correction are fundamental applications of the Levenshtein distance algorithm. The algorithm calculates the minimum number of edits (insertions, deletions, or substitutions) needed to transform one string into another. This makes it ideal for identifying misspelled words and suggesting corrections. Below, we demonstrate a practical implementation of a spell-checker in Python:
class SpellChecker:
def __init__(self, dictionary_words):
self.dictionary = set(word.lower() for word in dictionary_words)
def find_closest_words(self, word: str, max_distance: int = 2) -> list:
"""Find all dictionary words within max_distance of the input word."""
word = word.lower()
return [
(dict_word, distance)
for dict_word in self.dictionary
if (distance := levenshtein_distance(word, dict_word)[0]) <= max_distance
]
def suggest_corrections(self, word: str, max_suggestions: int = 5) -> list:
"""Suggest corrections for a potentially misspelled word."""
if word.lower() in self.dictionary:
return []
suggestions = self.find_closest_words(word)
# Sort by distance and limit number of suggestions
return sorted(suggestions, key=lambda x: (x[1], x[0]))[:max_suggestions]
# Example usage
dictionary = ["apple", "apples", "appeal", "appear", "appease"]
spell_checker = SpellChecker(dictionary)
misspelled_words = ["appel", "apple", "appl", "appels"]
for word in misspelled_words:
suggestions = spell_checker.suggest_corrections(word)
print(f"\nSuggestions for '{word}':")
for suggestion, distance in suggestions:
print(f" {suggestion} (distance: {distance})")
This implementation starts with a dictionary of known words, converts them to lowercase for uniformity, and uses the Levenshtein distance to calculate the similarity between the input word and dictionary words. It also ranks suggestions based on the edit distance, ensuring the most relevant suggestions appear first.
Here’s an example of the spell-checker in action:
Suggestions for 'appel': appeal (distance: 1) appear (distance: 2) apple (distance: 2) apples (distance: 2) Suggestions for 'apple': Suggestions for 'appl': apple (distance: 1) appeal (distance: 2) apples (distance: 2) Suggestions for 'appels': appeal (distance: 2) appear (distance: 2) appease (distance: 2) apple (distance: 2) apples (distance: 2)
Notice how the algorithm handles variations in spelling by providing ranked suggestions, ensuring users receive accurate and meaningful corrections.
2. Fuzzy String Matching for Database Deduplication
When working with large datasets, especially those containing user-entered or unstructured data, it's common to encounter duplicate records with slight variations. For example, company names might be formatted differently or contain minor spelling errors. Fuzzy string matching using Levenshtein distance helps identify and merge these duplicates effectively.
Below is a Python implementation of a DuplicateFinder
class that leverages the Levenshtein distance algorithm to identify duplicates based on a configurable similarity threshold:
@dataclass
class DuplicateMatch:
"""Represents a duplicate match between two strings."""
str1: str
str2: str
similarity: float
original_str1: str
original_str2: str
def __str__(self) -> str:
return f"Match ({self.similarity:.2%} similar):\n - {self.original_str1}\n - {self.original_str2}"
class DuplicateFinder:
"""
Finds duplicate strings using Levenshtein distance with configurable parameters.
"""
def __init__(
self,
similarity_threshold: float = 0.8,
case_sensitive: bool = False,
ignore_punctuation: bool = True,
ignore_whitespace: bool = True
):
if not 0 <= similarity_threshold <= 1:
raise ValueError("Similarity threshold must be between 0 and 1")
self.threshold = similarity_threshold
self.case_sensitive = case_sensitive
self.ignore_punctuation = ignore_punctuation
self.ignore_whitespace = ignore_whitespace
def normalize_string(self, s: str) -> str:
"""Normalize strings for comparison."""
normalized = s.lower() if not self.case_sensitive else s
if self.ignore_punctuation:
normalized = re.sub(r'[^\w\s]', '', normalized)
if self.ignore_whitespace:
normalized = ' '.join(normalized.split())
return normalized
def similarity_score(self, s1: str, s2: str) -> float:
"""Calculate similarity score between two strings."""
normalized_s1 = self.normalize_string(s1)
normalized_s2 = self.normalize_string(s2)
max_len = max(len(normalized_s1), len(normalized_s2))
if max_len == 0:
return 1.0
distance = levenshtein_distance(normalized_s1, normalized_s2)[0]
return 1 - (distance / max_len)
def find_duplicates(self, records: List[str]) -> List[DuplicateMatch]:
"""
Find potential duplicates in a list of strings.
"""
pairs = [(rec1, rec2)
for i, rec1 in enumerate(records)
for rec2 in records[i+1:]]
matches = []
for str1, str2 in pairs:
similarity = self.similarity_score(str1, str2)
if similarity >= self.threshold:
matches.append(DuplicateMatch(str1, str2, similarity, str1, str2))
return matches
# Example usage
if __name__ == "__main__":
company_names = [
"Microsoft Corporation",
"Microsoft Corp.",
"Microsoft Corp",
"MicroSoft Corporation",
"Apple Inc.",
"Apple Inc",
"Apple Incorporated",
"Alphabet Inc.",
"Google LLC"
]
finder = DuplicateFinder(
similarity_threshold=0.85,
case_sensitive=False,
ignore_punctuation=True,
ignore_whitespace=True
)
duplicates = finder.find_duplicates(company_names)
print("Potential duplicate companies:")
for match in duplicates:
print(f"\n{match}")
The DuplicateFinder
class normalizes strings by handling case sensitivity, punctuation, and whitespace, ensuring consistent comparisons. The similarity_score
method calculates the similarity between two strings using Levenshtein distance, and duplicates are identified based on the configured similarity threshold.
Here’s an example output of the DuplicateFinder
in action:
Potential duplicate companies: Match (100.00% similar): - Microsoft Corporation - MicroSoft Corporation Match (95.00% similar): - Microsoft Corp. - Microsoft Corp Match (95.00% similar): - Apple Inc. - Apple Inc
This implementation ensures scalability for large datasets and supports configurable options to adapt to various domains. It’s a powerful tool for cleaning and deduplicating records in databases.
3. DNA Sequence Alignment
In bioinformatics, the Levenshtein distance algorithm plays a vital role in analyzing DNA sequences. By calculating the number of edits required to transform one DNA sequence into another, we can identify mutations, insertions, and deletions. This analysis is particularly useful in studying genetic variations and identifying potential errors or mutations in DNA sequences.
The following Python implementation demonstrates a DNAAnalyzer
class that validates DNA sequences and analyzes mutations between two sequences:
class DNAAnalyzer:
def __init__(self):
self.valid_bases = set('ATCG')
def validate_sequence(self, sequence: str) -> bool:
"""Validate that a sequence contains only valid DNA bases."""
return all(base in self.valid_bases for base in sequence.upper())
def analyze_mutation(self, seq1: str, seq2: str) -> dict:
"""Analyze mutations between two DNA sequences."""
if not (self.validate_sequence(seq1) and self.validate_sequence(seq2)):
raise ValueError("Invalid DNA sequence")
distance, matrix = levenshtein_distance(seq1, seq2)
# Find specific mutations
mutations = []
i, j = len(seq1), len(seq2)
while i > 0 and j > 0:
if seq1[i-1] == seq2[j-1]:
i, j = i-1, j-1
else:
if matrix[i][j] == matrix[i-1][j-1] + 1:
mutations.append(('substitution', i-1, seq1[i-1], seq2[j-1]))
i, j = i-1, j-1
elif matrix[i][j] == matrix[i-1][j] + 1:
mutations.append(('deletion', i-1, seq1[i-1], '-'))
i -= 1
else:
mutations.append(('insertion', j-1, '-', seq2[j-1]))
j -= 1
return {
'distance': distance,
'similarity': 1 - (distance / max(len(seq1), len(seq2))),
'mutations': list(reversed(mutations))
}
# Example usage
dna_analyzer = DNAAnalyzer()
seq1 = "ACGTACGT"
seq2 = "ACGTATGT"
result = dna_analyzer.analyze_mutation(seq1, seq2)
print(f"Analyzing DNA sequences:")
print(f"Sequence 1: {seq1}")
print(f"Sequence 2: {seq2}")
print(f"\nResults:")
print(f"Edit distance: {result['distance']}")
print(f"Similarity: {result['similarity']:.2%}")
print("\nMutations:")
for mutation in result['mutations']:
m_type, pos, old, new = mutation
print(f"Position {pos}: {m_type} ({old} → {new})")
This implementation starts by validating that the input sequences contain only valid DNA bases (A, T, C, G). It calculates the Levenshtein distance and identifies mutations, including substitutions, insertions, and deletions, using the computed distance matrix. The method analyze_mutation
provides a detailed analysis of how one sequence differs from another.
Here’s an example output of the DNAAnalyzer
in action:
Analyzing DNA sequences: Sequence 1: ACGTACGT Sequence 2: ACGTATGT Results: Edit distance: 1 Similarity: 87.50% Mutations: Position 5: substitution (C → T)
This example shows how the algorithm identifies a substitution mutation (C → T) at position 5 in the DNA sequence, with an edit distance of 1 and a similarity score of 87.5%.
DNA sequence alignment is crucial in bioinformatics for comparing sequences, identifying mutations, and understanding genetic variations. The Levenshtein distance provides an efficient and reliable way to achieve this.
Relationship to Other String Distance Metrics
Levenshtein distance is one of several string metrics used for measuring the difference between sequences. Understanding its relationship to other metrics helps in choosing the right algorithm for specific use cases.
Related Distance Metrics
Metric | Key Characteristics | Main Differences from Levenshtein | Best Use Cases |
---|---|---|---|
Hamming Distance |
|
|
|
Damerau-Levenshtein |
|
|
|
Longest Common Subsequence (LCS) |
|
|
|
Jaro-Winkler |
|
|
|
Feature Comparison Matrix
Feature | Levenshtein | Hamming | Damerau-Levenshtein | LCS | Jaro-Winkler |
---|---|---|---|---|---|
Handles Different Lengths | ✓ | ✗ | ✓ | ✓ | ✓ |
Insertions/Deletions | ✓ | ✗ | ✓ | ✓ | ✓ |
Substitutions | ✓ | ✓ | ✓ | ✗ | ✓ |
Transpositions | ✗ | ✗ | ✓ | ✗ | ✓ |
Position Sensitivity | Position-based | Strict | Position-based | Sequential | Weighted |
Normalized Output | Manual | Manual | Manual | Manual | Built-in |
Computational Complexity | O(mn) | O(n) | O(mn) | O(mn) | O(mn) |
Space Complexity | O(min(m,n)) | O(1) | O(mn) | O(mn) | O(m+n) |
Key Insights
- Versatility vs. Specificity: Levenshtein strikes a balance between flexibility and complexity, handling different string lengths, insertions, deletions, and substitutions. In contrast, Hamming is specific to equal-length strings and only supports substitutions. Damerau-Levenshtein extends Levenshtein with transpositions, making it better for correcting human errors. Metrics like LCS focus on preserving sequence order, while Jaro-Winkler emphasizes prefix matching.
- Performance Tradeoffs: Simpler metrics like Hamming distance (O(n)) perform faster because they have fewer features but are limited to equal-length strings. More complex metrics like Damerau-Levenshtein (O(mn)) provide better accuracy with transpositions but are computationally heavier. Levenshtein and LCS also run in O(mn), making them suitable for moderate-sized datasets. Jaro-Winkler, while O(m+n), is optimized for short strings with weighted prefix matching.
- Feature Explanations and Use Cases:
Each feature in the comparison matrix aligns with specific strengths:
- Handles Different Lengths: Metrics like Levenshtein, Damerau-Levenshtein, LCS, and Jaro-Winkler handle strings of unequal lengths, while Hamming requires strings of equal length.
- Insertions/Deletions: Supported by Levenshtein, Damerau-Levenshtein, LCS, and Jaro-Winkler for flexible string comparison. Hamming cannot handle these operations.
- Substitutions: A core feature of Levenshtein, Hamming, Damerau-Levenshtein, and Jaro-Winkler. LCS does not support substitutions as it only tracks the longest sequence of matching characters.
- Transpositions: Only Damerau-Levenshtein and Jaro-Winkler handle adjacent transpositions, useful for typing errors. Levenshtein, Hamming, and LCS ignore transpositions.
- Position Sensitivity: - Levenshtein and Damerau-Levenshtein are position-sensitive, accounting for edits at specific positions. - LCS focuses on maintaining sequence order without substitutions. - Jaro-Winkler weights matches by position, favoring prefix alignment. - Hamming strictly enforces exact position matching.
- Normalized Output: Jaro-Winkler provides a built-in similarity score between 0 and 1. Other metrics, such as Levenshtein and Damerau-Levenshtein, require manual normalization (e.g., dividing by max string length) for similarity scoring.
- Computational and Space Complexity: Hamming has the best performance with O(n) time and O(1) space. Metrics like Levenshtein and Damerau-Levenshtein have higher computational costs (O(mn)) but are versatile. LCS also has O(mn) complexity, while Jaro-Winkler is faster for short strings with O(m+n) complexity.
- Use Case Alignment:
Each metric’s features align with specific scenarios:
- Levenshtein: General-purpose string comparison across domains like spell-checking and DNA sequence alignment.
- Hamming: Ideal for error detection in fixed-length codes and binary strings.
- Damerau-Levenshtein: Designed for human input correction, such as typo fixes in spell-checking and text processing.
- LCS: Best for sequence alignment in bioinformatics or file diff tools to compare ordered sequences.
- Jaro-Winkler: Optimized for name matching, record linkage, and comparing short strings with prefix emphasis.
Selection Guidelines
When choosing between string metrics, consider:
- Nature of the differences you expect (typographical errors, structural differences, etc.)
- Importance of position and order in the comparison
- Performance requirements and scale of implementation
- Need for normalized vs. absolute distance measures
- Domain-specific requirements (e.g., name matching, code comparison)
Conclusion
Throughout this guide, we've explored the mathematical foundations, implementations, and practical applications of the Levenshtein distance algorithm. From its origins in computational theory to modern applications in everything from spell-checking to bioinformatics, Levenshtein distance has proven to be a fundamental tool for measuring the similarity between strings through a clear, intuitive edit-distance approach.
Key Takeaways:
- Versatility: Levenshtein distance provides a robust foundation for string comparison, handling insertions, deletions, and substitutions with equal facility.
- Efficiency: With space-optimized implementations and clear complexity bounds, it scales effectively for most real-world applications.
- Implementation: The algorithm's straightforward dynamic programming approach makes it accessible and adaptable across programming languages and use cases.
As with any string metric, Levenshtein distance is most effective when applied thoughtfully and with consideration for your specific needs. Whether you're implementing fuzzy search, performing data deduplication, or analyzing genetic sequences, understanding both the capabilities and constraints of this algorithm will help you make informed decisions in your text processing applications.
If you found this guide helpful, please consider citing or sharing it with fellow developers and data scientists. For more resources on string metrics, implementation strategies, and practical applications, check out our Further Reading section.
Have fun and happy coding!
Further Reading
Core Concepts
-
Binary Codes Capable of Correcting Deletions, Insertions, and Reversals
Original 1966 paper by Vladimir Levenshtein introducing the distance metric and its mathematical foundations.
-
Jaro-Winkler Similarity: A Comprehensive Guide
Companion article exploring another important string similarity metric, with detailed comparisons and implementations.
-
Damerau-Levenshtein Distance: A Guide to String Similarity with Transpositions
Explores the extension of Levenshtein distance that accounts for transposition errors, making it particularly effective for spell checking and DNA sequence analysis.
-
Algorithms for Approximate String Matching
Comprehensive survey by Esko Ukkonen covering fundamental algorithms for string matching, including Levenshtein distance.
Implementation Resources
-
SymSpell Library
Efficient spell checking and string similarity library implementing Levenshtein distance with optimizations for large-scale applications.
-
RapidFuzz Documentation
Modern, fast library for string matching and comparison, with highly optimized Levenshtein distance implementation.
-
RapidFuzz C++
High-performance C++ implementation of string matching algorithms, including Levenshtein distance.
Advanced Applications
-
Fast String Matching for DNA Sequences
Recent research on optimizing string matching algorithms for genomic applications, including Levenshtein-based approaches.
-
Neural String Edit Distance
Novel approach combining Levenshtein distance with neural networks for improved string similarity measurement.
Performance Optimization
-
Efficient string similarity join in multi-core and distributed systems
Research on scalable implementations of string similarity metrics for big data applications.
Attribution and Citation
If you found this guide and tools helpful, feel free to link back to this page or cite it in your work!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.