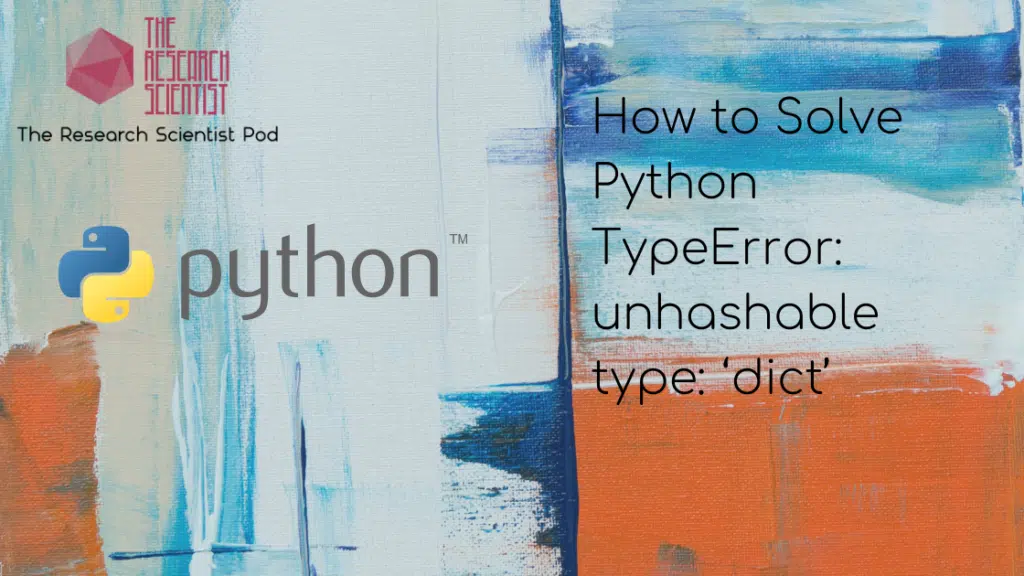
In Python, a dictionary is stores data in key:value pairs. Python 3.7 dictionaries are ordered data collections; Python 3.6 and previous dictionaries are unordered. In a Python dictionary, all keys must be hashable. If you try to use the unhashable key type ‘dict’ when adding a key or retrieving a value, you will raise the error “TypeError: unhashable type: ‘dict'”.
This tutorial will go through the error in detail, and we will go through an example scenario of the error and learn to solve it.
Table of contents
TypeError: unhashable type: ‘dict’
What Does TypeError Mean?
TypeError occurs whenever you try to perform an illegal operation for a specific data type object. For example, if you try to slice a dictionary as if it were a list, the dictionary type does not support slicing, and you will raise the error “TypeError: unhashable type: ‘slice‘”.
What Does Unhashable Mean?
By definition, a dictionary key needs to be hashable. An object is hashable if it has a hash value that remains the same during its lifetime. A hash value is an integer Python uses to compare dictionary keys while looking at a dictionary. When we add a new key:value pair to a dictionary, the Python interpreter generates a hash of the key. We can only hash particular objects in Python, like string or integers but not dictionaries. All immutable built-in objects in Python are hashable, and Mutable containers like dictionaries are not hashable. Let’s look at an example scenario where we try to obtain the hash value of a dictionary:
# Example dictionary
dictionary = {
"name":"John",
"age":28
}
print(type(dictionary))
print(hash(dictionary))
TypeError Traceback (most recent call last)
1 print(hash(dictionary))
TypeError: unhashable type: 'dict'
The code prints out the type to confirm the dictionary object is a ‘dict’ object. When we try to print the dictionary’s hash, we raise the error: “TypeError: unhashable type: ‘dict'”.
Let’s look at an example scenario.
Example: Generating a Dictionary Key with Another Dictionary
# Define list of dictionaries containing several fruits and amounts of each
fruits = [
{
"name":"apples", "left":4
},
{
"name":"pears", "left":9
},
{
"name":"bananas", "left":3
}
]
Next, we will construct a for loop that iterates over the list of fruits and selects for fruits with more than three left in stock. The fruits in stock will be added to the dictionary called fruits_more_than_three. We then print each fruit with more than three in stock to the console. Once the for loop has run, the fruits_more_than_three dictionary prints to the console.
# For loop over list of dictionaries containing fruits and amount left
for f in fruits:
if f["left"] ≻ 3:
fruits_more_than_three[f] = f["left"]
print(f'There are more than three of {f["name"]} left')
Let’s see what happens when we try to run the code:
TypeError Traceback (most recent call last)
1 for f in fruits:
2 if f["left"] ≻ 3:
3 fruits_more_than_three[f] = f["left"]
4 print(f'There are more than three of {f["name"]} left')
5
TypeError: unhashable type: 'dict'
The error occurs because we attempt to generate a dictionary key with another dictionary. The value of f is a dictionary from the list of fruits. If we try to add something to the fruits_more_than_three dictionary, we add a dictionary as a key. When we execute the if statement on apple, the interpreter sees:
fruits_more_than_three[{"name":"apples", "left":4}] = 4
Solution
To solve this problem, you can use f[“name”] as the name of the fruits_more_than_three dictionary key. Let’s look at the change in the revised code
# Fix by using the value associated with the key "name" instead of using the dictionary as the key.
for f in fruits:
if f["left"] ≻ 3:
fruits_more_than_three[f["name"]] = f["left"]
print(f'There are more than three of {f["name"]} left')
print(fruits_more_than_three)
There are more than three of apples left
There are more than three of pears left
{'apple': 4, 'pear': 9, 'apples': 4, 'pears': 9}
The code runs because we are using the name of each fruit as the key for fruits_more_than_three.
Summary
Congratulations on reading to the end of this tutorial! The error “TypeError: unhashable type: ‘dict'” occurs when you try to create an item in a dictionary using a dictionary as a key. Python dictionary is an unhashable object. Only immutable objects like strings, tuples, and integers can be used as a key in a dictionary because they remain the same during the object’s lifetime.
To solve this error, ensure you use hashable objects when creating or retrieving an item from a dictionary.
Go to the online courses page on Python to learn more about Python for data science and machine learning.
Have fun and happy researching!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.