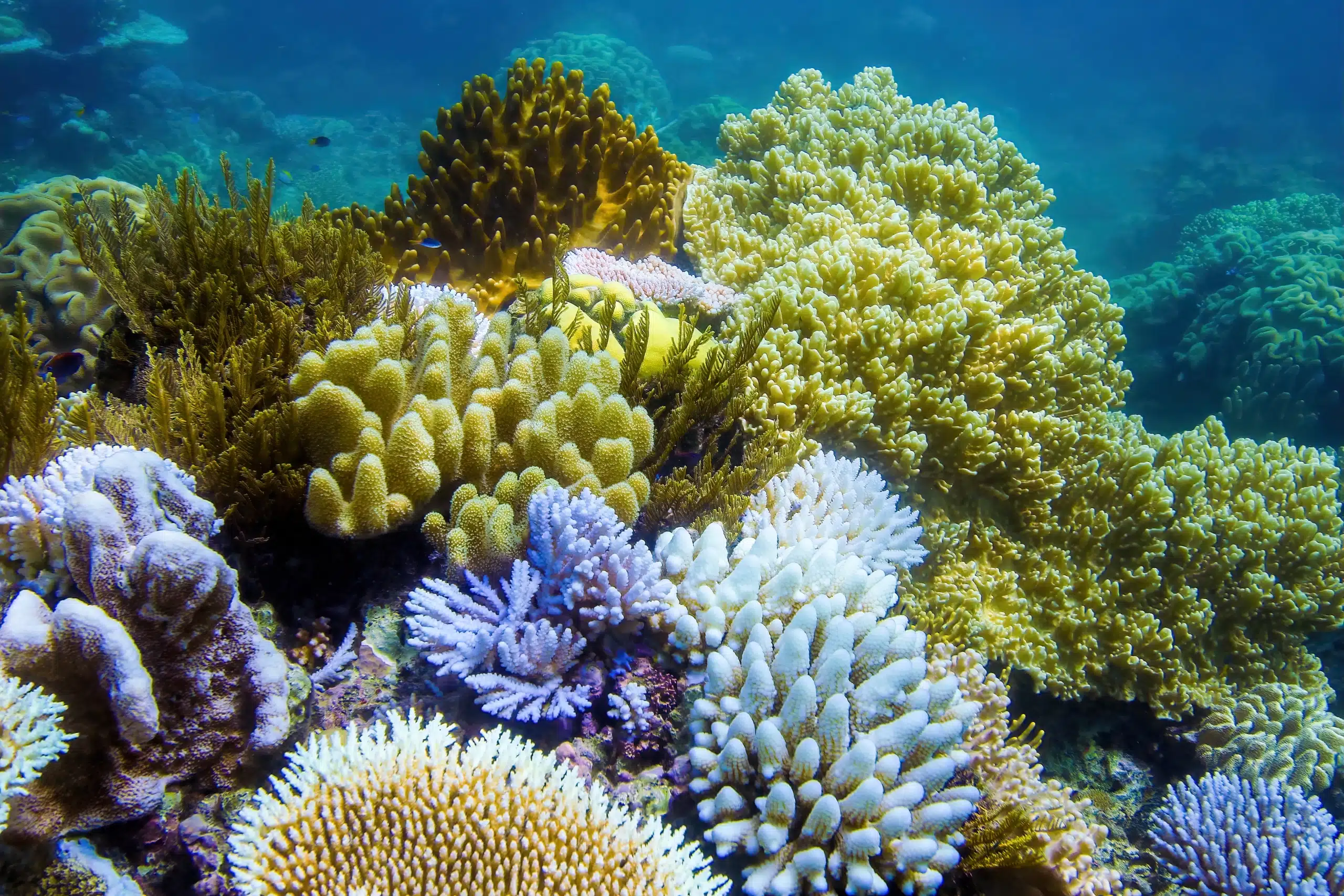
Simpson’s Diversity Index is a fundamental tool in ecological research that measures both species richness and evenness in a community. Unlike the Shannon’s Index, Simpson’s Index gives more weight to abundant species, making it particularly useful for understanding dominance patterns in ecosystems.
Table of Contents
Key Concepts
Simpson’s Index can be expressed in several forms:
1. Simpson’s Dominance Index (D):
\[ D = \sum_{i=1}^{s} p_i^2 \]2. Simpson’s Diversity Index (1-D):
\[ 1-D = 1 – \sum_{i=1}^{s} p_i^2 \]3. Simpson’s Reciprocal Index (1/D):
\[ \frac{1}{D} = \frac{1}{\sum_{i=1}^{s} p_i^2} \]where \(p_i\) is the proportion of individuals in species i, and s is the total number of species.
Conceptual Understanding
At its core, Simpson’s Index represents something remarkably intuitive: it’s the probability that two individuals randomly selected from a community will belong to the same species. This simple concept makes it one of the most meaningful and easily interpretable diversity measures available to ecologists.
The Probability Interpretation
Consider walking through a forest and randomly picking two leaves from the ground. Simpson’s Index answers the question: “What’s the chance these leaves came from the same type of tree?” In a forest dominated by a single species (low diversity), this probability would be high. In a forest with many equally common species (high diversity), this probability would be low.
Dominance vs. Diversity
The original Simpson’s Index (D) measures dominance:
- High Values (approaching 1): Indicate high probability of same-species draws, meaning the community is dominated by one or few species
- Low Values (approaching 0): Indicate low probability of same-species draws, meaning the community has many equally-abundant species
This creates an apparent paradox: higher values indicate lower diversity. To address this counter-intuitive scaling, ecologists commonly use two transformations:
- Simpson’s Diversity Index (1-D): Subtracts the probability from 1, so higher values now indicate higher diversity
- Simpson’s Reciprocal Index (1/D): Takes the inverse, creating a scale from 1 to the total number of species
Advantages of Simpson’s Index
- Sample Size Independence: Unlike many other diversity measures, Simpson’s Index is relatively robust to differences in sample size, making it reliable for comparing communities sampled with different intensities.
- Taxonomic Flexibility: The index’s focus on dominance patterns rather than absolute species identification makes it useful even when detailed taxonomic expertise is limited.
- Intuitive Interpretation: The probability-based definition provides a concrete, real-world interpretation that’s easy to understand and explain.
Interpretation Guidelines
• Simpson’s Dominance (D): Ranges from 0 to 1, higher values indicate lower diversity
• Simpson’s Diversity (1-D): Ranges from 0 to 1, higher values indicate higher diversity
• Simpson’s Reciprocal (1/D): Ranges from 1 to S (number of species), higher values indicate higher diversity
Example Calculation
Understanding Simpson’s Index is best achieved through practical application. Let’s work through a detailed example using data from a coral reef fish community survey. This example will demonstrate not only the mathematical process but also how to interpret the results in an ecological context. We’ll calculate all three forms of Simpson’s Index (D, 1-D, and 1/D) to show how they provide different perspectives on the same community structure.
In our survey, we’ve recorded four common reef fish species. The data represents the number of individuals counted during a standardized visual census along a 50-meter transect. While this is a simplified dataset, it illustrates the key principles that apply to more complex community analyses.
Coral Reef Fish Community
Species | Count | Proportion (pi) | pi² |
---|---|---|---|
Parrotfish | 40 | 0.400 | 0.160 |
Butterflyfish | 30 | 0.300 | 0.090 |
Damselfish | 20 | 0.200 | 0.040 |
Angelfish | 10 | 0.100 | 0.010 |
Calculations:
D = 0.160 + 0.090 + 0.040 + 0.010 = 0.300
1-D = 1 – 0.300 = 0.700
1/D = 1/0.300 = 3.333
Real-World Applications
Simpson’s Diversity Index is widely used in various ecological and environmental studies, including:
- Conservation Biology: Assessing biodiversity in ecosystems to prioritize conservation efforts.
- Forestry: Evaluating tree species diversity in different forest types.
- Agriculture: Measuring crop species diversity for sustainable farming.
- Marine Biology: Studying species dominance in coral reef systems and fisheries.
Understanding species diversity helps researchers identify ecosystems at risk and plan effective management strategies.
Implementation in Python
The calculation of Simpson’s diversity indices can be efficiently implemented using Python, particularly leveraging the NumPy library for numerical computations. The implementation below provides a reusable function that calculates all three common forms of Simpson’s Index (D, 1-D, and 1/D) in a single operation.
This implementation focuses on several key features:
- Vectorized operations using NumPy for improved performance with large datasets
- Robust handling of input data through automatic array conversion
- Clear documentation following scientific computing conventions
- Return of all common index forms in a single dictionary for convenience
The function takes a simple list or array of species abundances as input, making it easy to use with data from field surveys or experimental studies. While the example uses our coral reef data, the function works with any community abundance data, regardless of the taxonomic group or ecosystem type.
import numpy as np
def simpsons_diversity(abundances):
"""
Calculate Simpson's Diversity indices from species abundances.
Parameters:
-----------
abundances : array-like
List or array of species abundances
Returns:
--------
dict : Dictionary containing D, 1-D, and 1/D
"""
abundances = np.array(abundances)
total = abundances.sum()
proportions = abundances / total
D = np.sum(proportions ** 2)
return {
'D': D,
'1-D': 1 - D,
'1/D': 1 / D
}
# Example usage
abundances = [40, 30, 20, 10] # Our coral reef example
results = simpsons_diversity(abundances)
print(f"Simpson's Dominance (D): {results['D']:.3f}")
print(f"Simpson's Diversity (1-D): {results['1-D']:.3f}")
print(f"Simpson's Reciprocal (1/D): {results['1/D']:.3f}")
Simpson’s Dominance (D): 0.300
Simpson’s Diversity (1-D): 0.700
Simpson’s Reciprocal (1/D): 3.333
Implementation in R
R has long been the standard for ecological data analysis, and implementing Simpson’s diversity indices in R offers several advantages for ecological research. While specialized packages like ‘vegan’ provide pre-built diversity functions, understanding how to implement these calculations from scratch is valuable for both learning and customization purposes.
The R implementation below offers several key features particularly relevant to ecological data analysis:
- Built-in data cleaning with automatic removal of zero abundances
- Native R vectorization for efficient computation
- List-based return structure that matches R’s conventional data structures
- Easy integration with R’s extensive ecosystem of statistical and plotting packages
For more complex analyses, this basic implementation can be easily extended to work with R’s data frames, which are commonly used for ecological datasets containing multiple samples and associated metadata. The function accepts simple numeric vectors but can be modified to handle more complex data structures as needed.
simpsons_diversity <- function(abundances) {
# Convert to numeric and remove zeros
abundances <- as.numeric(abundances[abundances > 0])
# Calculate proportions
total <- sum(abundances)
proportions <- abundances / total
# Calculate D
D <- sum(proportions^2)
# Return all three indices
list(
D = D,
"1-D" = 1 - D,
"1/D" = 1 / D
)
}
# Example usage
abundances <- c(40, 30, 20, 10) # Our coral reef example
results <- simpsons_diversity(abundances)
# Print results
cat(sprintf("Simpson's Dominance (D): %.3f\n", results$D))
cat(sprintf("Simpson's Diversity (1-D): %.3f\n", results$`1-D`))
cat(sprintf("Simpson's Reciprocal (1/D): %.3f\n", results$`1/D`))
Simpson's Dominance (D): 0.300
Simpson's Diversity (1-D): 0.700
Simpson's Reciprocal (1/D): 3.333
Comparison with Shannon Index
While both Simpson's and Shannon's indices are fundamental tools in biodiversity measurement, they approach the challenge of quantifying diversity from distinctly different mathematical and conceptual foundations. Simpson's Index, rooted in probability theory, asks about the chances of encountering the same species twice, while Shannon's Index, derived from information theory, measures the uncertainty in predicting the species identity of a randomly sampled individual.
These different theoretical underpinnings lead to practical differences in how the indices behave and what they reveal about community structure. Simpson's Index is particularly sensitive to changes in the abundance of common species, making it excellent for detecting shifts in community dominance. In contrast, Shannon's Index provides a more balanced sensitivity to both rare and common species, making it better suited for tracking overall community changes.
Understanding these complementary strengths and limitations is crucial for choosing the appropriate index for specific research questions. Let's examine key differences between these indices across several important dimensions:
Metric | Simpson's Index | Shannon Index |
---|---|---|
Focus | Abundance and dominance; emphasizes common species | Richness and evenness; balances rare and common species |
Weighting | Squares proportional abundances (p²), giving more weight to abundant species | Uses natural logarithm (p × ln(p)), giving more balanced species weights |
Range | • D: 0 to 1 (dominance) • 1-D: 0 to 1 (diversity) • 1/D: 1 to S (reciprocal) |
• H: 0 to ln(S) • Typically 1.5 to 3.5 in real communities • J (evenness): 0 to 1 |
Sensitivity | Less sensitive to rare species; better for detecting changes in abundant species | More sensitive to rare species; better for detecting overall community changes |
Sample Size Dependence | Relatively sample-size independent; good for comparing different-sized samples | More sensitive to sample size; requires similar sampling effort for valid comparisons |
Interpretation | Probability-based interpretation (chance of selecting same species twice); more intuitive | Information theory-based interpretation (uncertainty in species identity); more abstract |
Best Use | • Ecosystems with dominant species • Monitoring changes in dominant species • Comparing communities with different sample sizes • When intuitive interpretation is needed |
• General biodiversity studies • Detecting changes in rare species • When both common and rare species are important • Long-term monitoring programs |
Calculation Complexity | Simpler calculations; easier to compute by hand | More complex calculations; typically requires calculator or computer |
Choosing Between Indices
The choice between Simpson's and Shannon's indices often depends on:
- Study objectives (focus on dominant vs. rare species)
- Sampling constraints (different vs. standardized sample sizes)
- Need for intuitive interpretation
- Type of ecosystem being studied
Many researchers choose to report both indices to provide complementary perspectives on community diversity.
Conclusion
Simpson's Diversity Index is a simple yet reliable tool for measuring species dominance in ecological research. Its three forms (D, 1-D, and 1/D) offer flexibility, while its low sensitivity to sample size makes it ideal for comparing communities.
By focusing on dominant species, Simpson's Index complements other measures like the Shannon Index, which balances species richness and evenness. Together, these tools provide a clearer picture of community structure and ecosystem health. In ecological research, no single index is sufficient—using multiple measures ensures a more complete understanding of biodiversity.
Further Reading
-
Simpson Diversity Calculator
Our dedicated Simpson Index calculator.
-
Shannon Diversity Calculator
Our dedicated calculator helps you compute Shannon Diversity Index and Equitability.
-
Shannon Diversity Index Guide
Explore the connections between Shannon's Index and other diversity measures in ecological research.
-
Simpson Index
A fundamental paper explaining Simpson's index.
-
A conceptual guide to measuring species diversity
This paper advocates for using coverage-based sampling and Hill diversity metrics to obtain more robust and comparable estimates of species diversity in ecological studies, addressing the limitations of traditional methods like species richness, Shannon index, and Simpson index.
-
Sørensen-Dice Coefficient: A Comprehensive Guide
Master similarity measurement with the Sørensen-Dice coefficient, including implementations in Python and R, applications in medical imaging, text analysis, and ecological research.
-
Bray-Curtis Dissimilarity: A Comprehensive Guide
Master ecological distance measurement with Bray-Curtis dissimilarity, including Python and R implementations, applications in community ecology, biodiversity assessment, and environmental monitoring.
Attribution and Citation
If you found this guide and tools helpful, feel free to link back to this page or cite it in your work!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.