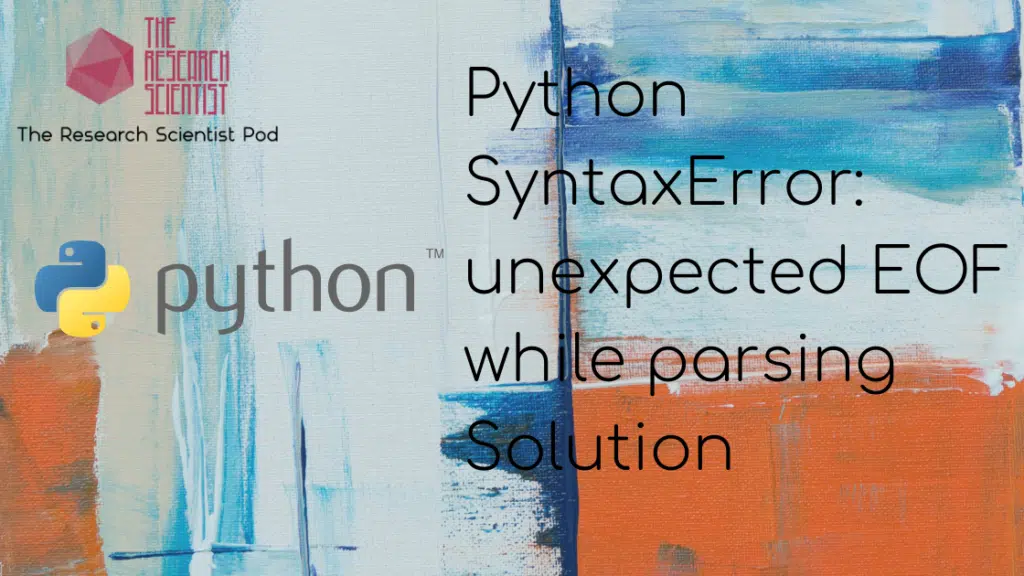
“SyntaxError: unexpected EOF while parsing” occurs when the program finishes abruptly before executing all of the code. This error can happen when you make a structural or syntax mistake in the code, most likely a missing parenthesis or an incorrectly indented block.
SyntaxError tells us that we broke one of the syntax rules to follow when writing a Python program. If we violate any Python syntax, the Python interpreter will raise a SyntaxError. A common example of this error is “SyntaxError: unexpected character after line continuation character“. This error occurs when you add code after using a line continuation character, which violates the syntax rules for line continuation.
Another common error of this type is “SyntaxError: can’t assign to function call“, which occurs when variable assignment using the response of a function call is done in the wrong order.
EOF in the error message stands for End of File or the last character in your program. In this tutorial, we will go through the possible causes of this error, including:
- Not enclosing the code inside a special statement, for example, a for loop
- Not closing all of the opening parentheses in the code
- Unfinished try statement
Table of contents
Example 1: Not Enclosing Code In Special Statement
Special statements in Python include for loops, if-else statements, and while loops, among others, require at least one line of code in their statement. The code will specify a task to perform within the constraints of the statement. If we do not include the line of code inside the special statement, we will raise an unexpected EOF error. Let’s look at an example of iterating over a list using a for loop:
string_list = ['do', 'or', 'do', 'not', 'there', 'is', 'no', 'try']
for string in string_list:
File "<ipython-input-2-2844f36d2179>", line 1
for string in string_list:
^
SyntaxError: unexpected EOF while parsing
The following code results in an error because there is no indented code block within the “for loop”. Because there is no indented code, Python does not know where to end the statement, raising the SyntaxError. To fix this, we need to add a line of code, for example, a print() statement, to get the individual strings in the list.
for string in string_list:
print(string)
do
or
do
not
.
there
is
no
try
The code prints each word in the list of words, meaning we have resolved the SyntaxError.
We may not want to add any meaningful code within the special statement. In this instance, we can add a “pass” statement, telling the code not to do anything within the loop.
for string in string_list:
pass
We can include the “pass” statement when we want a placeholder while focusing on developing the structure of a program. Once we establish the structure, we can replace the placeholder with the actual code.
Python indentation tells a Python interpreter that a group of statements belongs to a particular block of code. We can understand blocks of code as groupings of statements for specific purposes. Other languages like C, C++, and Java use braces “{ }” to define a block of code, and we can indent further to the right to nest a block more deeply.
Example 2: Not Closing an Open Parenthesis
If you forget to close all the opening parentheses within a line of code, the Python interpreter will raise the SyntaxError. Let’s look at an example of an unclosed print statement.”
print("He who controls the spice, controls the universe."
File "<ipython-input-7-eb7ac88cf989>", line 1
print("He who controls the spice, controls the universe."
^
SyntaxError: unexpected EOF while parsing
The print statement is missing a closing parenthesis on the right-hand side. The SyntaxError trace indicates where the missing parenthesis is, and we can add a closing parenthesis where hinted.
print("He who controls the spice, controls the universe.")
He who controls the spice, controls the universe.
Missing parentheses are coming occurrences. Fortunately, many Integrated Developer Environments (IDEs) like PyCharm and advanced text editors like Visual Studio Code automatically produce the opening and closing parenthesis. These tools also provide syntax highlighting and the different parenthesis pairings in your code, which can help significantly reduce the frequency SyntaxErrors. In a related article, I discuss the benefit of IDEs and advanced text editors and the best data science and machine learning specific libraries in Python.
Example 3: Unfinished Try Statement
You can use try statements for exception handling, but you need to pair them with at least except or finally clause. If you try to run an isolated try statement, you will raise a SyntaxError, as shown:
try:
print("Welcome to Arrakis")
File "<ipython-input-10-5eb175f61894>", line 2
print("Welcome to Arrakis")
^
SyntaxError: unexpected EOF while parsing
The caret indicates the source of the error. To handle exceptions, we can add either an “except” or a “finally” clause.
try:
print("Welcome to Arrakis")
except:
print("Not successful")
Welcome to Arrakis
try:
print("Welcome to Arrakis")
finally:
print("Thank you")
Welcome to Arrakis
Thank you
We can use the “except” statement for when an exception occurs. We can use the “finally” clause, which will always run, even if an exception occurs. Both clauses solve the SyntaxError.
Example 4: Using eval() function on str()
Python does not allow for using the eval() function on str(), and the interpreter will raise the SyntaxError. eval() is a built-in function that parses the expression argument and evaluates it as a Python expression. In other words, the function evaluates the “string” as a Python expression and returns the result as an integer. Developers tend to use the eval() function for evaluating mathematical expressions. Let’s look at an example of using the eval() function on str()
string_1 = 'this is a string'
string_2 = eval(str(string_1))
if string_1 == string_2:
print("eval() works!")
File "<ipython-input-14-220b2a2edc6b>", line 1, in <module>
string_2 = eval(str(string_1))
File "<string>", line 1
this is a string
^
SyntaxError: unexpected EOF while parsing
To avoid the SyntaxError, we can replace the str() function with the repr() function, which produces a string that holds a printable representation of an object.
string_1 = 'this is a string'
string_2 = eval(repr(string_1))
if string_1 == string_2:
print("eval() works!")
eval() works
Summary
Congratulations on reaching the end of this tutorial! You know what causes this common SyntaxError and how to solve it. SyntaxError: unexpected EOF, while parsing occurs when Python abruptly reaches the end of execution before executing every code line. Specifically, we will see this error when:
- The code has an incomplete special statement, for example, an if statement.
- There is incorrect or no indentation
- An unclosed opening parenthesis.
- An isolated try block with no “finally” or “except” clauses.
- Trying to implement eval() on str().
To avoid this error, ensure your code has complete statements, proper indentation, which you can resolve automatically by using an IDE or advanced text editor for development. Also, ensure you define an except or finally clause if the code has a try statement.
You are ready to solve this SyntaxError like a pro! If you want to learn about other common errors, you can find errors such as Python ValueError: invalid literal for int() with base 10. If you want to learn more about Python for data science and machine learning, visit the online courses page.
Have fun and happy researching!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.