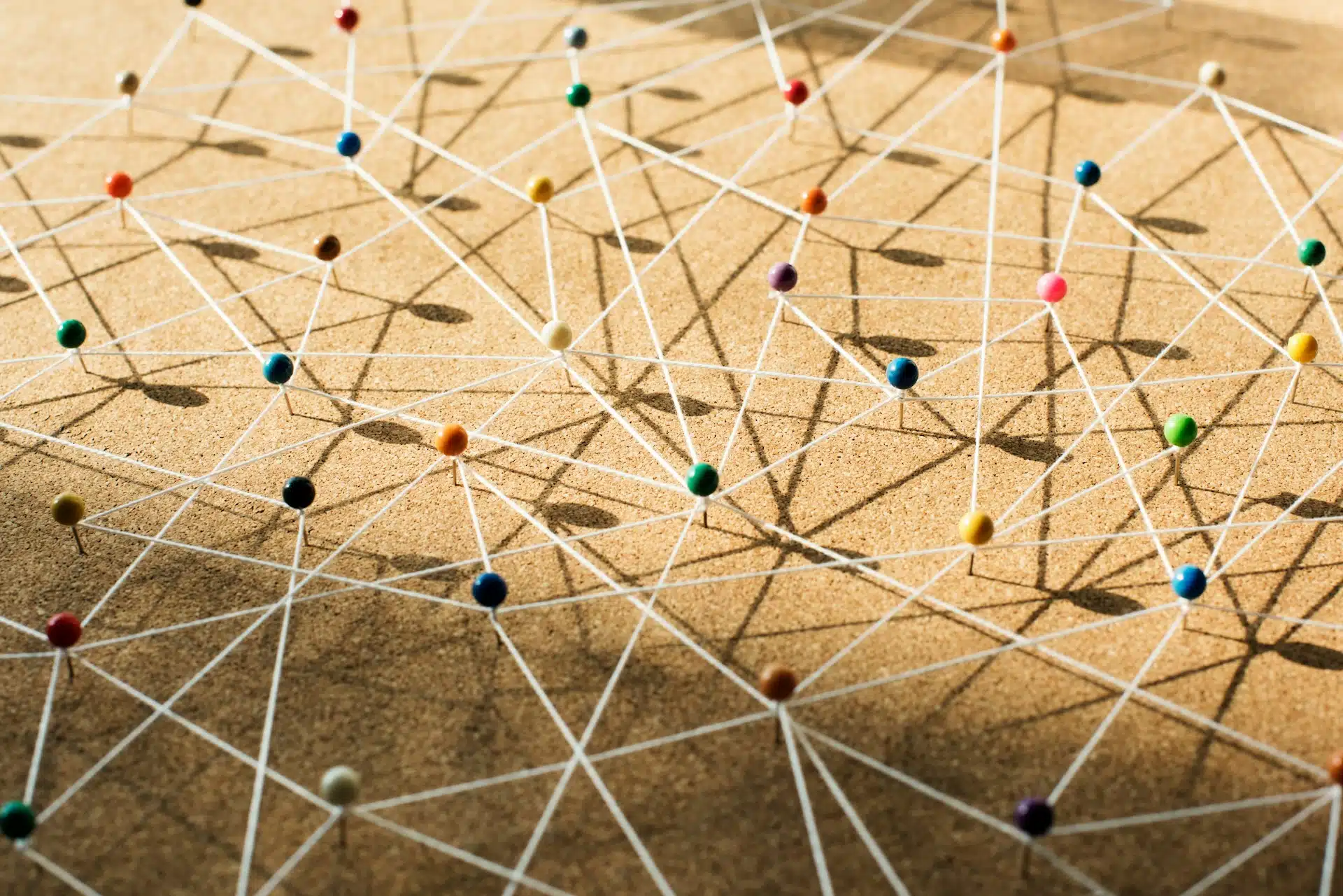
Table of Contents
Introduction
The Bellman-Ford algorithm solves one of graph theory’s most intriguing challenges: finding shortest paths from a single source vertex to all others, even when some edges carry negative weights. While Dijkstra’s algorithm falters with negative edges, Bellman-Ford thrives, making it invaluable for applications from routing protocols to financial arbitrage detection.
Key Features of Bellman-Ford
- Negative Weight Handling: Uniquely capable of processing graphs with negative edge weights
- Cycle Detection: Can identify negative cycles that would make shortest paths undefined
- Robustness: Guarantees shortest paths in the absence of negative cycles
- Simplicity: Uses a straightforward iterative approach
Core Concept: Edge Relaxation
At its heart, Bellman-Ford works through a process called edge relaxation. Think of each edge as a path that can be improved – like finding a better route on your daily commute. The algorithm methodically examines each possible path, updating the shortest known distances as it discovers improvements.
How Edge Relaxation Works
For each edge (u,v) with weight w:
- Check if we can improve the path to v by going through u
- If distance[u] + w < distance[v], update distance[v]
- Repeat this process |V|-1 times, where |V| is the number of vertices
Algorithm Overview
# Bellman-Ford Algorithm - High-Level Overview
1. Initialize distances:
for each vertex v in V:
distance[v] = ∞
distance[source] = 0
2. Relax edges repeatedly:
for i from 1 to |V| - 1:
for each edge (u, v) with weight w:
if distance[u] + w < distance[v]:
distance[v] = distance[u] + w
predecessor[v] = u
3. Check for negative-weight cycles:
for each edge (u, v) with weight w:
if distance[u] + w < distance[v]:
report "Graph contains a negative-weight cycle"
4. Return distances and predecessors
Iteration | A | B | C | D | E |
---|---|---|---|---|---|
Distance | 0 | ∞ | ∞ | ∞ | ∞ |
Predecessor | - | - | - | - | - |
Real-World Applications
Bellman-Ford's unique ability to handle negative weights opens doors to solving complex real-world problems:
- Networking: Powers routing protocols like RIP (Routing Information Protocol), helping data find efficient paths through the internet
- Financial Systems: Enables detection of arbitrage opportunities in currency exchange markets
- Resource Allocation: Manages systems with both costs and benefits, represented as positive and negative weights
- Network Timing: Facilitates clock synchronization in distributed systems
Important Considerations
While Bellman-Ford offers unique capabilities, it comes with certain trade-offs:
- Time complexity of O(|V|·|E|) vs Dijkstra's O((|V|+|E|) log |V|)
- Required for negative weights but slower for non-negative weights
- Can detect negative cycles, crucial for many applications
In the following sections, we'll explore the mathematical foundations and implementation details that make Bellman-Ford a reliable choice for tackling complex pathfinding challenges. We'll examine how it compares with other algorithms and discover where it proves most valuable in practice.
Try it Yourself: Interactive Pathfinding Visualizer
Experiment with the Bellman-Ford algorithm in action! Generate random mazes, visualize the pathfinding process, and compare different algorithms including Dijkstra's, A*, Bellman-Ford, BFS, and DFS. Features real-time animations and path-related statistics.
🧠 Pathfinding Showdown: Algorithm Comparison
Watch five powerful algorithms race through intricate mazes in this interactive visualization that showcases their unique search patterns and efficiency with beautiful animations and real-time metrics. Compare how Dijkstra's methodical approach differs from A*'s heuristic-guided search, BFS's level-by-level exploration, DFS's deep diving technique, and Bellman-Ford's ability to handle complex weight scenarios.
The visualization demonstrates real-time statistics including nodes visited, path length, execution time, and memory usage, allowing you to directly observe the efficiency tradeoffs between these algorithms in action.
Mathematical Background
The Bellman-Ford algorithm builds on a fundamental principle: systematically improving path estimates through successive iterations. Let's explore the mathematical framework that makes this possible.
Formal Definition
Consider a weighted graph \(G = (V, E)\) where:
- \(V = \{v_1, v_2, ..., v_n\}\): The set of vertices
- \(E \subset V \times V\): The set of edges
- \(w: E \to \mathbb{R}\): The weight function mapping edges to real numbers
The initial state is defined as:
\[ distance[v] = \begin{cases} 0 & \text{if } v \text{ is the source} \\ \infty & \text{otherwise} \end{cases} \]
Edge Relaxation
Edge relaxation forms the core operation. For an edge from u to v with weight w:
If \(distance[u] + w < distance[v]\), then:
\[distance[v] = distance[u] + w\]
Iteration Bound: Why |V|-1?
The algorithm performs |V|-1 iterations because:
- Any shortest path is a simple path (no cycles)
- A simple path contains at most |V|-1 edges
- After k iterations, we've found shortest paths using ≤ k edges
Negative Cycles and Their Applications
A negative cycle occurs when the sum of edge weights around a cycle is negative. This concept has practical applications, particularly in financial markets.
Currency Exchange Example
To illustrate, consider currency exchanges where each conversion is represented as a graph edge. For example:
- Start: You begin with 1000 USD.
-
Convert USD to EUR:
Multiply 1000 USD by 0.85:
\(1000 \, \text{USD} \times 0.85 = 850 \, \text{EUR}\) -
Convert EUR to GBP:
Multiply 850 EUR by 0.88:
\(850 \, \text{EUR} \times 0.88 = 748 \, \text{GBP}\) -
Convert GBP back to USD:
Multiply 748 GBP by 1.4:
\(748 \, \text{GBP} \times 1.4 = 1047.20 \, \text{USD}\)
The conversion results in a net gain, indicating an arbitrage opportunity. To analyze this using Bellman-Ford, we transform each exchange rate using the formula:
Weight transformation:
\[w(\text{edge}) = -\ln(\text{exchange rate})\]
- USD → EUR: \(-\ln(0.85) \approx 0.163\)
- EUR → GBP: \(-\ln(0.88) \approx 0.128\)
- GBP → USD: \(-\ln(1.4) \approx -0.336\)
Summing these values:
\(0.163 + 0.128 + (-0.336) = -0.045\)
The negative sum indicates a negative cycle, hence an arbitrage opportunity.
Properties of Negative Cycles
- Shortest paths become undefined
- Path costs can be arbitrarily reduced
- Detection occurs after |V|-1 iterations
Applications of Negative Cycles
- Financial Markets: Currency arbitrage, pricing inconsistencies
- Resource Allocation: Task scheduling, supply chain optimization
- Network Routing: Loop detection, QoS optimization
Negative Cycle Detection
After |V|-1 iterations, check:
For each edge (u,v):
If \(distance[u] + w(u,v) < distance[v]\)
This works because:
- All valid shortest paths are found after |V|-1 iterations
- Further improvements imply a cycle
- A relaxable edge after |V|-1 iterations indicates a negative cycle
Implementation Considerations
- Include negative cycle detection
- Track cycle paths when needed
- Handle floating-point arithmetic carefully
- Use appropriate precision for financial calculations
Complexity Analysis
Time complexity: \(O(|V| \cdot |E|)\)
- Main phase: (|V|-1) iterations × |E| edges
- Detection phase: One pass through |E| edges
Summary
- Edge relaxation iteratively improves path estimates
- |V|-1 iterations guarantee optimal paths if no negative cycles exist
- Negative cycles indicate unlimited improvement potential
- Higher complexity than Dijkstra's algorithm but handles negative weights
Implementation
In this section, we'll implement the Bellman-Ford algorithm using a specific example graph. The implementations will demonstrate the algorithm's ability to handle negative edge weights and detect negative cycles.
Example Graph Visualization
Graph Properties
- Vertices: A, B, C, D, E
- Edges: 10 directed edges with varying weights
- Negative edges: B→D (-4), E→C (-3), C→B (-2)
- No negative cycles present
- Source vertex: A
Expected Results
From source vertex A:
- A → A: 0
- A → B: 2 (via A → E → C → B)
- A → C: 4 (via A → E → C)
- A → D: -2 (via A → E → C → B → D)
- A → E: 7 (via A → E)
Let's implement the Bellman-Ford algorithm in various programming languages to solve this specific example. Each implementation will demonstrate the complete algorithm, including negative cycle detection and path reconstruction.
Python Implementation
Implements Bellman-Ford with path reconstruction and negative cycle detection.
from typing import Dict, List, Tuple, Optional
from math import inf
def bellman_ford(graph: Dict[str, List[Tuple[str, int]]], source: str) -> Tuple[Dict[str, int], Dict[str, Optional[str]]]:
# Initialize distances and predecessors
distance = {vertex: inf for vertex in graph}
predecessor = {vertex: None for vertex in graph}
distance[source] = 0
# Relax edges |V|-1 times
vertices = list(graph.keys())
for _ in range(len(vertices) - 1):
for u in graph:
for v, weight in graph[u]:
if distance[u] + weight < distance[v]:
distance[v] = distance[u] + weight
predecessor[v] = u
# Check for negative cycles
for u in graph:
for v, weight in graph[u]:
if distance[u] + weight < distance[v]:
raise ValueError("Graph contains a negative-weight cycle")
return distance, predecessor
def get_path(predecessor: Dict[str, Optional[str]], target: str) -> List[str]:
path = []
current = target
while current is not None:
path.append(current)
current = predecessor[current]
return path[::-1]
# Example graph from our visualization
graph = {
'A': [('B', 6), ('E', 7)],
'B': [('C', 5), ('D', -4), ('E', 8)],
'C': [('B', -2)],
'D': [('A', 2), ('C', 7)],
'E': [('C', -3), ('D', 9)]
}
try:
# Find shortest paths from source vertex 'A'
distances, predecessors = bellman_ford(graph, 'A')
# Print results
print("Shortest distances from A:")
for vertex in distances:
path = get_path(predecessors, vertex)
print(f"A → {vertex}: {distances[vertex]} (Path: {' → '.join(path)})")
except ValueError as e:
print(f"Error: {e}")
# Expected output:
#Shortest distances from A:
#A → A: 0 (Path: A)
#A → B: 2 (Path: A → E → C → B)
#A → C: 4 (Path: A → E → C)
#A → D: -2 (Path: A → E → C → B → D)
#A → E: 7 (Path: A → E)
Java Implementation
Uses Java collections and proper encapsulation for the algorithm.
import java.util.*;
public class BellmanFord {
private static class Edge {
String source, target;
int weight;
Edge(String source, String target, int weight) {
this.source = source;
this.target = target;
this.weight = weight;
}
}
private static class PathInfo {
Map<String, Integer> distances;
Map<String, String> predecessors;
PathInfo(Map<String, Integer> distances, Map<String, String> predecessors) {
this.distances = distances;
this.predecessors = predecessors;
}
}
public static PathInfo findShortestPaths(List<String> vertices, List<Edge> edges, String source) {
// Initialize distances and predecessors
Map<String, Integer> distances = new HashMap<>();
Map<String, String> predecessors = new HashMap<>();
for (String vertex : vertices) {
distances.put(vertex, Integer.MAX_VALUE);
predecessors.put(vertex, null);
}
distances.put(source, 0);
// Relax edges |V|-1 times
for (int i = 0; i < vertices.size() - 1; i++) {
for (Edge edge : edges) {
if (distances.get(edge.source) != Integer.MAX_VALUE &&
distances.get(edge.source) + edge.weight < distances.get(edge.target)) {
distances.put(edge.target, distances.get(edge.source) + edge.weight);
predecessors.put(edge.target, edge.source);
}
}
}
// Check for negative cycles
for (Edge edge : edges) {
if (distances.get(edge.source) != Integer.MAX_VALUE &&
distances.get(edge.source) + edge.weight < distances.get(edge.target)) {
throw new IllegalArgumentException("Graph contains a negative-weight cycle");
}
}
return new PathInfo(distances, predecessors);
}
public static List<String> getPath(Map<String, String> predecessors, String target) {
List<String> path = new ArrayList<>();
String current = target;
while (current != null) {
path.add(0, current);
current = predecessors.get(current);
}
return path;
}
public static void main(String[] args) {
// Create example graph
List<String> vertices = Arrays.asList("A", "B", "C", "D", "E");
List<Edge> edges = Arrays.asList(
new Edge("A", "B", 6), new Edge("A", "E", 7),
new Edge("B", "C", 5), new Edge("B", "D", -4), new Edge("B", "E", 8),
new Edge("C", "B", -2),
new Edge("D", "A", 2), new Edge("D", "C", 7),
new Edge("E", "C", -3), new Edge("E", "D", 9)
);
try {
PathInfo result = findShortestPaths(vertices, edges, "A");
System.out.println("Shortest distances from A:");
for (String vertex : vertices) {
List<String> path = getPath(result.predecessors, vertex);
System.out.printf("A → %s: %d (Path: %s)%n",
vertex, result.distances.get(vertex),
String.join(" → ", path));
}
} catch (IllegalArgumentException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
/* Expected Output:
Shortest distances from A:
A → A: 0 (Path: A)
A → B: 2 (Path: A → E → C → B)
A → C: 4 (Path: A → E → C)
A → D: -2 (Path: A → E → C → B → D)
A → E: 7 (Path: A → E)*/
JavaScript Implementation
Uses modern JavaScript features with clear error handling and path reconstruction.
class BellmanFord {
constructor(vertices) {
this.vertices = vertices;
this.graph = new Map();
// Initialize graph structure
vertices.forEach(vertex => {
this.graph.set(vertex, []);
});
}
addEdge(source, target, weight) {
this.graph.get(source).push({ target, weight });
}
findShortestPaths(source) {
// Initialize distances and predecessors
const distances = new Map();
const predecessors = new Map();
this.vertices.forEach(vertex => {
distances.set(vertex, Infinity);
predecessors.set(vertex, null);
});
distances.set(source, 0);
// Relax edges |V|-1 times
for (let i = 0; i < this.vertices.length - 1; i++) {
this.vertices.forEach(u => {
this.graph.get(u).forEach(({ target: v, weight }) => {
if (distances.get(u) + weight < distances.get(v)) {
distances.set(v, distances.get(u) + weight);
predecessors.set(v, u);
}
});
});
}
// Check for negative cycles
this.vertices.forEach(u => {
this.graph.get(u).forEach(({ target: v, weight }) => {
if (distances.get(u) + weight < distances.get(v)) {
throw new Error("Graph contains a negative-weight cycle");
}
});
});
return { distances, predecessors };
}
getPath(predecessors, target) {
const path = [];
let current = target;
while (current !== null) {
path.unshift(current);
current = predecessors.get(current);
}
return path;
}
}
// Example usage with our graph
try {
// Create graph
const vertices = ['A', 'B', 'C', 'D', 'E'];
const bf = new BellmanFord(vertices);
// Add edges
bf.addEdge('A', 'B', 6);
bf.addEdge('A', 'E', 7);
bf.addEdge('B', 'C', 5);
bf.addEdge('B', 'D', -4);
bf.addEdge('B', 'E', 8);
bf.addEdge('C', 'B', -2);
bf.addEdge('D', 'A', 2);
bf.addEdge('D', 'C', 7);
bf.addEdge('E', 'C', -3);
bf.addEdge('E', 'D', 9);
// Find shortest paths from 'A'
const { distances, predecessors } = bf.findShortestPaths('A');
console.log("Shortest distances from A:");
vertices.forEach(vertex => {
const path = bf.getPath(predecessors, vertex);
console.log(
`A → ${vertex}: ${distances.get(vertex)} ` +
`(Path: ${path.join(' → ')})`
);
});
} catch (error) {
console.error("Error:", error.message);
}
/* Expected Output:
Shortest distances from A:
A → A: 0 (Path: A)
A → B: 2 (Path: A → E → C → B)
A → C: 4 (Path: A → E → C)
A → D: -2 (Path: A → E → C → B → D)
A → E: 7 (Path: A → E)*/
C++ Implementation
Implements Bellman-Ford with STL containers and RAII principles.
#include <iostream>
#include <vector>
#include <unordered_map>
#include <limits>
#include <string>
#include <stdexcept>
class BellmanFord {
private:
struct Edge {
std::string source, target;
int weight;
Edge(std::string s, std::string t, int w)
: source(s), target(t), weight(w) {}
};
std::vector<std::string> vertices;
std::vector<Edge> edges;
public:
BellmanFord(const std::vector<std::string>& v) : vertices(v) {}
void addEdge(const std::string& source,
const std::string& target,
int weight) {
edges.emplace_back(source, target, weight);
}
struct PathInfo {
std::unordered_map<std::string, int> distances;
std::unordered_map<std::string, std::string> predecessors;
};
PathInfo findShortestPaths(const std::string& source) {
PathInfo result;
const int INF = std::numeric_limits<int>::max();
// Initialize distances and predecessors
for (const auto& vertex : vertices) {
result.distances[vertex] = INF;
result.predecessors[vertex] = "";
}
result.distances[source] = 0;
// Relax edges |V|-1 times
for (size_t i = 0; i < vertices.size() - 1; ++i) {
for (const auto& edge : edges) {
if (result.distances[edge.source] != INF &&
result.distances[edge.source] + edge.weight <
result.distances[edge.target]) {
result.distances[edge.target] =
result.distances[edge.source] + edge.weight;
result.predecessors[edge.target] = edge.source;
}
}
}
// Check for negative cycles
for (const auto& edge : edges) {
if (result.distances[edge.source] != INF &&
result.distances[edge.source] + edge.weight <
result.distances[edge.target]) {
throw std::runtime_error(
"Graph contains a negative-weight cycle");
}
}
return result;
}
std::vector<std::string> getPath(
const std::unordered_map<std::string, std::string>& predecessors,
const std::string& target) {
std::vector<std::string> path;
std::string current = target;
while (!current.empty()) {
path.insert(path.begin(), current);
auto it = predecessors.find(current);
if (it == predecessors.end()) break;
current = it->second;
}
return path;
}
};
int main() {
try {
// Create example graph
std::vector<std::string> vertices = {"A", "B", "C", "D", "E"};
BellmanFord bf(vertices);
// Add edges
bf.addEdge("A", "B", 6);
bf.addEdge("A", "E", 7);
bf.addEdge("B", "C", 5);
bf.addEdge("B", "D", -4);
bf.addEdge("B", "E", 8);
bf.addEdge("C", "B", -2);
bf.addEdge("D", "A", 2);
bf.addEdge("D", "C", 7);
bf.addEdge("E", "C", -3);
bf.addEdge("E", "D", 9);
// Find shortest paths from 'A'
auto result = bf.findShortestPaths("A");
std::cout << "Shortest distances from A:\n";
for (const auto& vertex : vertices) {
auto path = bf.getPath(result.predecessors, vertex);
std::cout << "A → " << vertex << ": "
<< result.distances[vertex] << " (Path: ";
// Print path
for (size_t i = 0; i < path.size(); ++i) {
if (i > 0) std::cout << " → ";
std::cout << path[i];
}
std::cout << ")\n";
}
}
catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
/* Expected Output:
Shortest distances from A:
A → A: 0 (Path: A)
A → B: 2 (Path: A → E → C → B)
A → C: 4 (Path: A → E → C)
A → D: -2 (Path: A → E → C → B → D)
A → E: 7 (Path: A → E)
*/
Implementation Notes
- All implementations use consistent graph representation
- Include both path reconstruction and negative cycle detection
- Provide clear error handling for negative cycles
- Output identical results for our example graph
Performance Considerations
- Time Complexity: O(|V| · |E|)
- Space Complexity: O(|V|) for distance and predecessor storage
- Each implementation uses appropriate data structures for its language
- Path reconstruction adds O(|V|) space but provides valuable path information
Relationship to Other Path-Finding Algorithms
The Bellman–Ford algorithm differs from other shortest path algorithms in several key ways:
Algorithm | Relationship to Bellman–Ford | Key Differences | Trade-offs |
---|---|---|---|
Dijkstra's Algorithm |
|
|
|
A* Algorithm |
|
|
|
Floyd-Warshall |
|
|
|
Algorithm Selection Guidelines
- Use Bellman-Ford when:
- Graph contains negative edge weights
- Need to detect negative cycles
- Single-source paths are needed
- Graph is relatively sparse
- Use Dijkstra's when:
- All edge weights are non-negative
- Need fastest single-source solution
- Memory efficiency is important
- Use A* when:
- Finding path to specific target
- Have good heuristic available
- Edge weights are non-negative
- Speed is critical
- Use Floyd-Warshall when:
- Need all-pairs shortest paths
- Graph is dense
- Memory constraints allow O(V²) storage
Feature Comparison Matrix
The matrix below provides a comprehensive comparison of key features and characteristics across major pathfinding algorithms:
Feature | Bellman–Ford | Dijkstra's | A* | Floyd-Warshall |
---|---|---|---|---|
Time Complexity | O(VE) | O((V+E) log V) | O(b^d)* | O(V³) |
Space Complexity | O(V) | O(V) | O(b^d) | O(V²) |
Negative Weights | ✓ | ✗ | ✗ | ✓ |
Negative Cycle Detection | ✓ | ✗ | ✗ | Partial** |
Single-Source | ✓ | ✓ | ✗ | ✗ |
Single-Target | ✗ | ✗ | ✓ | ✗ |
All-Pairs | ✗ | ✗ | ✗ | ✓ |
Heuristic Guided | ✗ | ✗ | ✓ | ✗ |
Optimal Solution | ✓ | ✓ | ✓*** | ✓ |
Memory Usage | Low | Medium | High | Very High |
Implementation Complexity | Medium | Medium | High | Low |
- * Where b is branching factor and d is solution depth
- ** Floyd-Warshall requires additional checks for negative cycles
- *** A* is optimal only with admissible and consistent heuristics
Algorithm Selection Guidelines
Algorithm | Best Used When | Avoid When | Common Applications |
---|---|---|---|
Bellman-Ford |
|
|
|
Dijkstra's |
|
|
|
A* |
|
|
|
Floyd-Warshall |
|
|
|
Selection Considerations:
- Performance requirements should be carefully evaluated
- Memory constraints may limit algorithm choice
- Consider graph properties (density, edge weights)
- Application-specific requirements may dictate choice
Conclusion
The Bellman–Ford algorithm provides a robust solution for finding shortest paths in graphs that include negative weight edges. Its ability to detect negative cycles makes it invaluable in many applications, despite its higher time complexity compared to algorithms like Dijkstra’s.
When to Use Bellman–Ford
- When your graph contains negative weight edges.
- When you need to detect the presence of negative cycles.
- In routing protocols where edge weights can vary.
Implementation Considerations
- Ensure proper initialization of distances and predecessor pointers.
- Iterate exactly |V| - 1 times for relaxation.
- Always perform an extra iteration to check for negative cycles.
Best Practices
- Validate input graphs for consistency.
- Optimize inner loops for performance in large graphs.
- Use the algorithm as a building block for more complex routing or optimization problems.
With its simplicity and broad applicability, the Bellman–Ford algorithm remains a key tool in the algorithmic toolbox.
If you found this guide helpful, please consider citing or sharing it. For additional resources on graph algorithms, check out our Further Reading section.
Happy pathfinding!
Core Concepts
-
Shortest Paths in Graphs
A classic paper discussing methods for shortest path calculations, including early ideas behind the Bellman–Ford algorithm.
-
Relaxation and Negative Cycle Detection
A detailed lecture on the concepts behind edge relaxation and cycle detection in graphs.
-
Data Structures and Algorithms (DSA) - Complete Guide
A comprehensive collection of solutions and implementations for all fundamental algorithms and data structures. Includes detailed tutorials on sorting algorithms (bubble sort, merge sort, quick sort), searching algorithms (binary search, linear search), tree traversals, graph algorithms, and more. Serves as a central hub linking to in-depth explanations and implementations for each topic.
-
Dijkstra's Algorithm: A Comprehensive Guide to Single-Source Shortest Paths
Complete guide to understanding Dijkstra's algorithm, which forms the foundation of A*. Includes detailed explanations, implementations, and visualizations of the base algorithm that A* extends.
-
A* Algorithm and Its Relationship to Dijkstra's Algorithm: A Comprehensive Guide to Intelligent Pathfinding
A detailed guide to understanding and implementing the A* (A-star) algorithm for intelligent pathfinding. This resource compares A*’s heuristic-driven approach with other common pathfinding algorithms, covering theoretical concepts, pseudocode, mathematical foundations, and implementations in Python, Java, C++, and JavaScript.
-
Floyd–Warshall Algorithm: A Comprehensive Guide to All-Pairs Shortest Paths
An in-depth guide to the Floyd–Warshall algorithm, covering its theoretical foundations, dynamic programming approach, and ability to compute all-pairs shortest paths efficiently. This guide includes pseudocode, mathematical background, performance analysis, and step-by-step implementations in Python, Java, C++, and JavaScript. Additionally, it explores detecting negative cycles and practical applications in network routing, computational geometry, and graph analysis.
-
Wikipedia: Bellman–Ford Algorithm
In-depth description, mathematical details, and historical context.
Implementation Resources
-
HappyCoders.eu Pathfinding Algorithm Tutorials and Implementations
GitHub repository covering Bellman–Ford along with other shortest path algorithms with links to tutorials.
-
HackerEarth Tutorials on Shortest Path Algorithms
Tutorials and coding challenges covering Bellman–Ford along with other shortest path algorithms.
Advanced Topics
-
Negative Cycle Detection and Applications
Research exploring advanced applications and improvements to the Bellman–Ford approach.
-
Optimizations and Parallel Implementations
A study on modern optimizations, parallel versions, and practical enhancements of the algorithm.
-
Princeton University Lecture Notes on Bellman–Ford
University-level lecture notes that provide a comprehensive look at graph algorithms, including Bellman–Ford.
Attribution and Citation
If you found this guide and tools helpful, feel free to link back to this page or cite it in your work!
Suf is a senior advisor in data science with deep expertise in Natural Language Processing, Complex Networks, and Anomaly Detection. Formerly a postdoctoral research fellow, he applied advanced physics techniques to tackle real-world, data-heavy industry challenges. Before that, he was a particle physicist at the ATLAS Experiment of the Large Hadron Collider. Now, he’s focused on bringing more fun and curiosity to the world of science and research online.